react路由守卫怎么实现
时间: 2024-01-30 12:46:57 浏览: 58
在 React 中实现路由守卫可以使用 React Router 提供的 `Route` 组件和 `history` 对象。
首先,需要使用 `Route` 组件来定义路由。可以根据需要设置 `path`、`component`、`exact` 等属性。例如:
```jsx
import { Route } from 'react-router-dom';
<Route path="/user" component={User} exact />
```
然后,需要使用 `history` 对象来控制路由跳转。可以通过 `withRouter` HOC 包装组件,来获取 `history` 对象。
```jsx
import { withRouter } from 'react-router-dom';
class User extends React.Component {
handleLogout = () => {
// 执行退出登录操作
// ...
// 跳转到登录页
this.props.history.push('/login');
}
render() {
// ...
}
}
export default withRouter(User);
```
在 `handleLogout` 方法中,可以执行退出登录操作,并使用 `history` 对象跳转到登录页。
最后,可以结合条件判断来实现路由守卫。例如:
```jsx
import { Redirect } from 'react-router-dom';
class App extends React.Component {
state = {
isLogin: true,
}
render() {
const { isLogin } = this.state;
return (
<div>
<Route path="/user" component={User} exact />
<Route path="/login" component={Login} exact />
{!isLogin && <Redirect to="/login" />}
</div>
);
}
}
```
在 `App` 组件中,可以根据条件判断是否需要跳转到登录页。如果用户未登录,就使用 `Redirect` 组件来跳转到登录页。注意,`Redirect` 组件必须放在 `Route` 组件的外面。
阅读全文
相关推荐
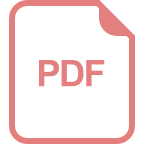
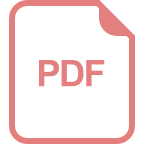








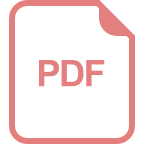
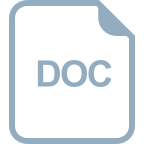
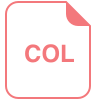




