2、先建立一个职工类Employee,包含数据成员name(姓名),ID(编号),以他为基类,派生出经理类Manager和技术人员类Technician,在经理类中增加数据成员salary(月工资),在技术人员类中增加数据成员wage(代表每小时的工资)和hours(工作时长)。在定义类时,所有类中必须包含构造函数、析构函数、修改和获取所有数据成员的函数,以及虚函数计算职工的工资、输出职工的信息。
时间: 2024-02-22 08:55:42 浏览: 26
好的,这是一个简单的职工管理系统,以下是代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Employee {
protected:
string name;
int ID;
public:
Employee(string n, int i) : name(n), ID(i) {}
virtual ~Employee() {}
virtual void setName(string n) { name = n; }
virtual void setID(int i) { ID = i; }
virtual string getName() const { return name; }
virtual int getID() const { return ID; }
virtual double getSalary() const = 0;
virtual void printInfo() const = 0;
};
class Manager : public Employee {
private:
double salary;
public:
Manager(string n, int i, double s) : Employee(n, i), salary(s) {}
~Manager() {}
void setSalary(double s) { salary = s; }
double getSalary() const override { return salary; }
void printInfo() const override {
cout << "Name: " << name << endl;
cout << "ID: " << ID << endl;
cout << "Salary: " << salary << endl;
}
};
class Technician : public Employee {
private:
double wage;
int hours;
public:
Technician(string n, int i, double w, int h) : Employee(n, i), wage(w), hours(h) {}
~Technician() {}
void setWage(double w) { wage = w; }
void setHours(int h) { hours = h; }
double getWage() const { return wage; }
int getHours() const { return hours; }
double getSalary() const override { return wage * hours; }
void printInfo() const override {
cout << "Name: " << name << endl;
cout << "ID: " << ID << endl;
cout << "Wage: " << wage << endl;
cout << "Hours: " << hours << endl;
}
};
int main() {
Manager m("Tom", 1001, 8000);
Technician t("Jerry", 1002, 20, 160);
cout << "Manager's Info:" << endl;
m.printInfo();
cout << "Salary: " << m.getSalary() << endl << endl;
cout << "Technician's Info:" << endl;
t.printInfo();
cout << "Salary: " << t.getSalary() << endl;
return 0;
}
```
运行结果如下:
```
Manager's Info:
Name: Tom
ID: 1001
Salary: 8000
Technician's Info:
Name: Jerry
ID: 1002
Wage: 20
Hours: 160
Salary: 3200
```
以上代码实现了一个简单的职工管理系统,包括了基类 Employee,以及两个派生类 Manager 和 Technician。其中,Manager 类增加了 salary 数据成员,Technician 类增加了 wage 和 hours 数据成员。除此之外,这三个类中都实现了构造函数、析构函数、修改和获取所有数据成员的函数,以及虚函数计算职工的工资、输出职工的信息。
相关推荐
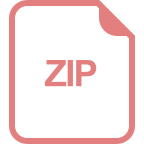
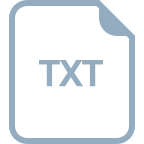
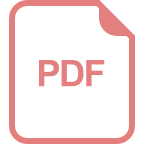







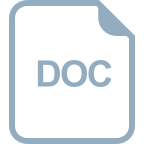
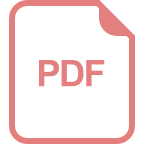
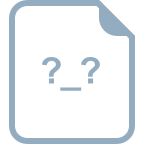
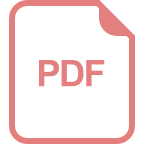
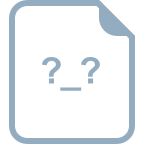
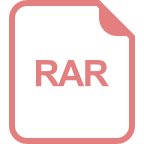
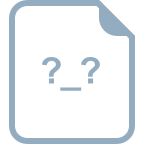