linux多线程编程
时间: 2023-07-11 22:06:57 浏览: 67
Linux多线程编程是指在Linux操作系统中使用多个线程来同时执行不同的任务,以提高程序的运行效率和响应速度。Linux提供了丰富的多线程编程接口,其中最常用的就是POSIX线程(Pthread)库。
使用Pthread库进行多线程编程的一般步骤如下:
1. 包含头文件pthread.h
2. 创建线程,使用pthread_create函数
3. 等待线程结束,使用pthread_join函数
4. 退出线程,使用pthread_exit函数
5. 销毁线程,使用pthread_cancel函数
下面是一个简单的例子,用于创建两个线程,分别输出“Hello”和“World”:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *print_hello(void *arg)
{
printf("Hello\n");
pthread_exit(NULL);
}
void *print_world(void *arg)
{
printf("World\n");
pthread_exit(NULL);
}
int main(int argc, char *argv[])
{
pthread_t t1, t2;
// 创建线程1
if (pthread_create(&t1, NULL, print_hello, NULL) != 0)
{
printf("Create thread 1 error!\n");
exit(1);
}
// 创建线程2
if (pthread_create(&t2, NULL, print_world, NULL) != 0)
{
printf("Create thread 2 error!\n");
exit(1);
}
// 等待线程1结束
if (pthread_join(t1, NULL) != 0)
{
printf("Join thread 1 error!\n");
exit(1);
}
// 等待线程2结束
if (pthread_join(t2, NULL) != 0)
{
printf("Join thread 2 error!\n");
exit(1);
}
return 0;
}
```
在上面的例子中,我们定义了两个函数print_hello和print_world,分别用于输出“Hello”和“World”。在主函数中,我们创建了两个线程t1和t2,分别执行print_hello和print_world函数。使用pthread_join函数等待两个线程结束,最后退出程序。
除了Pthread库之外,Linux还提供了其他多线程编程接口,如OpenMP、OpenCL等。不同的接口适用于不同的场景,需要根据具体情况选择合适的接口。
相关推荐
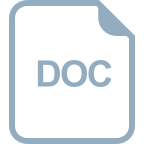














