react 前端滑动验证码组件
时间: 2023-07-09 08:29:27 浏览: 50
可以使用第三方库 React Swipeable,它提供了一个 Swipeable 组件,可以使用触摸操作进行滑动。结合 Canvas 可以实现滑动验证码功能。
具体实现步骤如下:
1. 安装 React Swipeable:`npm install react-swipeable --save`
2. 创建 Swipeable 组件,并添加 onTouchEnd 事件监听:
```jsx
import React from 'react';
import Swipeable from 'react-swipeable';
class SwipeableCanvas extends React.Component {
handleSwipe = () => {
// 滑动成功,执行相应操作
}
render() {
return (
<Swipeable onSwiped={this.handleSwipe}>
<canvas width="300" height="150"></canvas>
</Swipeable>
);
}
}
export default SwipeableCanvas;
```
3. 在 handleSwipe 方法中,获取 canvas 上下文并绘制验证码:
```jsx
handleSwipe = () => {
const canvas = document.getElementsByTagName('canvas')[0];
const ctx = canvas.getContext('2d');
const text = 'ABCDEFGHJKMNPQRSTWXYZabcdefhijkmnprstwxyz2345678';
// 绘制背景
ctx.fillStyle = '#f5f5f5';
ctx.fillRect(0, 0, canvas.width, canvas.height);
// 绘制文字
ctx.font = 'bold 50px Arial';
for (let i = 0; i < 4; i++) {
const char = text.charAt(Math.floor(Math.random() * text.length));
ctx.fillStyle = `rgb(${Math.random() * 255},${Math.random() * 255},${Math.random() * 255})`;
ctx.fillText(char, i * 70 + 20, 80);
}
}
```
4. 在 Swipeable 组件中添加 onTouchMove 事件监听,实现滑动效果:
```jsx
handleTouchMove = (e) => {
e.preventDefault();
const canvas = document.getElementsByTagName('canvas')[0];
const ctx = canvas.getContext('2d');
// 获取 canvas 左边距离页面左边的距离
const canvasLeft = canvas.getBoundingClientRect().left;
// 获取触摸点在 canvas 中的坐标
const touchX = e.changedTouches[0].clientX - canvasLeft;
const touchY = e.changedTouches[0].clientY - canvas.offsetTop;
// 绘制滑动轨迹
ctx.beginPath();
ctx.moveTo(this.lastX, this.lastY);
ctx.lineTo(touchX, touchY);
ctx.strokeStyle = '#000';
ctx.lineWidth = 5;
ctx.stroke();
this.lastX = touchX;
this.lastY = touchY;
}
handleSwipe = () => {
// 判断滑动是否成功,执行相应操作
}
handleTouchStart = (e) => {
const canvas = document.getElementsByTagName('canvas')[0];
// 获取 canvas 左边距离页面左边的距离
const canvasLeft = canvas.getBoundingClientRect().left;
// 获取触摸点在 canvas 中的坐标
this.lastX = e.changedTouches[0].clientX - canvasLeft;
this.lastY = e.changedTouches[0].clientY - canvas.offsetTop;
}
render() {
return (
<Swipeable
onSwiped={this.handleSwipe}
onSwiping={this.handleTouchMove}
onSwipingStart={this.handleTouchStart}
>
<canvas width="300" height="150"></canvas>
</Swipeable>
);
}
```
这样就可以实现一个基本的滑动验证码组件。需要注意的是,为了减少作弊行为,还需要在后端进行相应的验证。
阅读全文
相关推荐
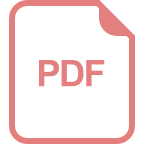
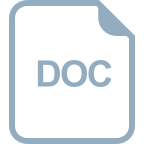

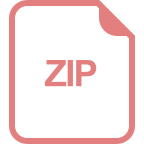
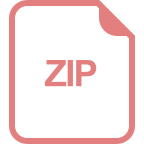
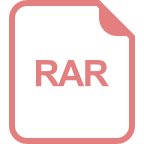
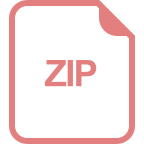
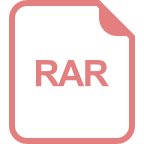
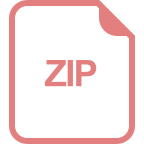
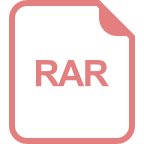
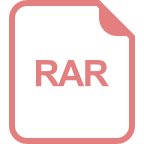
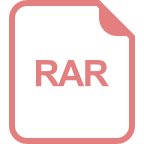
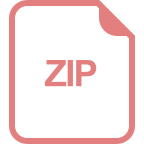
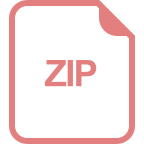