java读取excel,删除其中的A列为空的行,并将空行上移
时间: 2024-04-08 15:33:51 浏览: 80
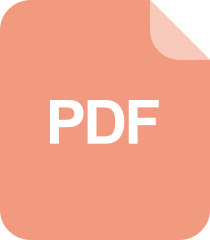
java如何读取Excel简单模板
要删除Excel中A列为空的行并将空行上移,你可以使用Apache POI库来读取和操作Excel文件。以下是一个示例代码,它将删除A列为空的行并将非空行上移一个位置:
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelUtils {
public static void removeEmptyRows(String filePath) {
try (FileInputStream fis = new FileInputStream(filePath);
Workbook workbook = new XSSFWorkbook(fis)) {
Sheet sheet = workbook.getSheetAt(0);
int lastRowNum = sheet.getLastRowNum();
for (int i = lastRowNum; i >= 0; i--) {
Row row = sheet.getRow(i);
Cell cell = row.getCell(0); // Assuming A列为第一列,下标为0
if (cell == null || cell.getCellType() == CellType.BLANK) {
sheet.shiftRows(i + 1, lastRowNum + 1, -1); // 上移非空行
sheet.removeRow(row);
}
}
try (FileOutputStream fos = new FileOutputStream(filePath)) {
workbook.write(fos);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
你可以调用 `ExcelUtils.removeEmptyRows(filePath)` 方法来删除指定Excel文件中A列为空的行,并将非空行上移一个位置。请确保在调用该方法之前,你已经安装了Apache POI库,并导入了相关的类。
阅读全文
相关推荐
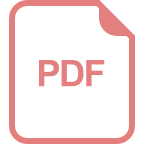
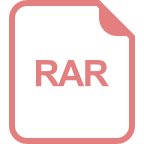















