编写C#程序定义一个类,描述一个矩形,包含有长、宽两种属性,和计算面积方法。 (1)编写一个类,继承自矩形类,长方体,具有长、宽、高属性,和计算体积的方法。 对以上两个类进行测试,创建一个长方体,定义其长、宽、高,输出其底面积和体积。
时间: 2024-03-11 07:51:40 浏览: 79
好的,以下是一个示例C#程序,实现了上述要求:
```csharp
using System;
// 定义矩形类
class Rectangle
{
public double length; // 长
public double width; // 宽
// 构造函数
public Rectangle(double length, double width)
{
this.length = length;
this.width = width;
}
// 计算面积的方法
public double CalculateArea()
{
return length * width;
}
}
// 定义长方体类,继承自矩形类
class Cuboid : Rectangle
{
public double height; // 高
// 构造函数
public Cuboid(double length, double width, double height) : base(length, width)
{
this.height = height;
}
// 计算体积的方法
public double CalculateVolume()
{
return length * width * height;
}
}
// 测试程序
class Program
{
static void Main(string[] args)
{
// 创建长方体对象
Cuboid cuboid = new Cuboid(2.0, 3.0, 4.0);
Console.WriteLine("长方体:长 = {0}, 宽 = {1}, 高 = {2}", cuboid.length, cuboid.width, cuboid.height);
Console.WriteLine("底面积 = {0}, 体积 = {1}", cuboid.CalculateArea(), cuboid.CalculateVolume());
Console.ReadKey();
}
}
```
这个程序定义了一个`Rectangle`矩形类,包含了`length`和`width`两个属性,以及`CalculateArea`方法用于计算面积;然后定义了`Cuboid`长方体类,继承自矩形类,增加了一个`height`属性,以及`CalculateVolume`方法用于计算体积。程序通过创建长方体对象,演示了类的继承关系和方法调用。
阅读全文
相关推荐
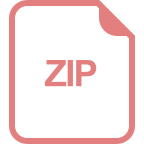
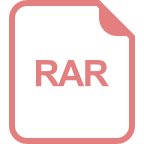
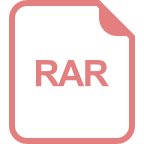
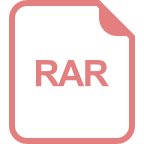
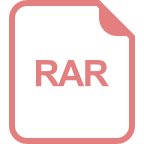
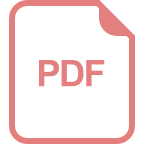
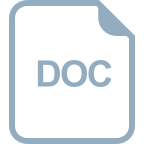
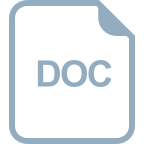
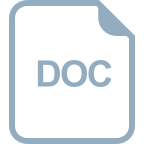
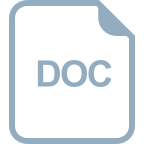
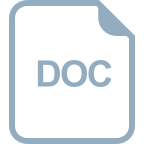
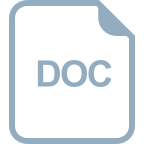
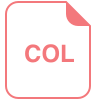
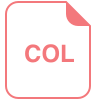