输入一个日期,输出这个日期是这一年的第几天,包括闰年的情况,用python实现,
时间: 2023-05-26 12:07:53 浏览: 62
以下是一个实现这个功能的Python程序:
```python
def is_leap(year):
"""判断是否为闰年"""
return year % 4 == 0 and (year % 100 != 0 or year % 400 == 0)
def day_of_year(date):
"""计算这一年中的第几天"""
year, month, day = map(int, date.split('-'))
days_in_month = [
31, 28 + is_leap(year), 31,
30, 31, 30,
31, 31, 30,
31, 30, 31
] # 每个月的天数
return sum(days_in_month[:month-1]) + day
date = input("请输入一个日期(格式为YYYY-MM-DD): ")
print("这一天是这一年的第{}天。".format(day_of_year(date)))
```
这个程序定义了两个函数:
- `is_leap`:判断给定年份是否为闰年(使用了闰年的定义);
- `day_of_year`:计算给定日期在这一年中是第几天,使用 `sum` 函数累加所在月份前面所有月份的天数即可。
输入一个日期后,会输出这个日期在这一年中的天数。
相关问题
输入一个日期,输出这个日期是这一年的第几天,用python实现
您可以使用datetime库中的datetime对象和timedelta对象来实现:
```python
from datetime import datetime
date_str = input("请输入日期,格式为yyyy-mm-dd: ")
date = datetime.strptime(date_str, "%Y-%m-%d")
day_of_year = (date - datetime(date.year, 1, 1)).days + 1
print("{0}是{1}年的第{2}天。".format(date_str, date.year, day_of_year))
```
代码解释:
1. 首先导入datetime库中的datetime对象
2. 接收用户输入的日期字符串
3. 将日期字符串转换成datetime对象
4. 使用timedelta对象计算该日期距离该年1月1日有几天
5. 将结果加1,输出即为该日期是该年的第几天
注意:该方法不适用于闰年。
编写程序从键盘输入一个日期,要求计算输出该日期是这一年的第几天
### 回答1:
以下是Python代码实现:
```python
date_str = input("请输入日期(格式为YYYY-MM-DD):")
year, month, day = map(int, date_str.split("-"))
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
days_in_month[1] = 29 # 闰年2月有29天
total_days = sum(days_in_month[:month-1]) + day
print(f"{year}年{month}月{day}日是这一年的第{total_days}天。")
```
代码解释:
1. 首先从键盘输入日期字符串,使用`split`方法将其拆分为年、月、日三个整数。
2. 定义一个列表`days_in_month`,存储每个月的天数。默认情况下2月有28天。
3. 判断输入的年份是否为闰年,如果是,则将`days_in_month`列表中2月的天数改为29。
4. 计算输入日期是这一年的第几天,即前面所有月份的天数之和加上当前月份的天数。
5. 使用`print`函数输出结果。
示例输出:
```
请输入日期(格式为YYYY-MM-DD):2022-03-15
2022年3月15日是这一年的第74天。
```
### 回答2:
本题的解决方案需要借助闰年和平年的概念,以及通过程序从键盘输入一个日期,并将其分离为年、月、日的数字。
首先,根据闰年和平年的规则,闰年指的是能够被4整除同时不能被100整除,或者是能够被400整除的年份;平年则为不符合这一条件的年份。
其次,要求将从键盘输入的日期拆分为年、月、日三个数字,可以使用字符串切片的方法将日期字符串划分为子字符串,然后通过类型转换将其转换为整型数值。例如,假设输入的日期格式为YYYY-MM-DD,则可以使用以下代码来分离日期:
```
date_str = input("请输入日期,格式为YYYY-MM-DD:")
year = int(date_str[:4])
month = int(date_str[5:7])
day = int(date_str[8:])
```
最后,根据每个月的天数以及闰平年的规则,可以编写程序计算出该日期是这一年的第几天。计算公式为:
```
if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:
days_per_month = [31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
else:
days_per_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
total_days = sum(days_per_month[:month-1]) + day
print("该日期是这一年的第{}天。".format(total_days))
```
在以上代码中,通过判断年份是否为闰年来确定每个月的天数列表(days_per_month),然后使用列表切片的方式将该月之前的所有天数相加,并加上当月的天数,即为该日期是这一年的第几天。
以上是编写程序从键盘输入一个日期,计算输出该日期是这一年的第几天的方法介绍和实现代码。
### 回答3:
编写程序实现从键盘输入一个日期,可以按照以下步骤实现:
1. 首先,提示用户输入一个日期,要求日期格式为“年-月-日”,例如“2022-08-01”。
2. 接着,为了提取出日期中的年、月和日,可以使用字符串的切割(substring)功能,或者使用正则表达式的匹配(match)功能。具体实现方法取决于编程语言。
3. 提取出年、月和日之后,可以通过以下公式计算出该日期是这一年的第几天:
```
day_of_year = day + sum(days_in_month[1:month-1])
```
其中,`day`表示日期中的日,`month`表示日期中的月,`days_in_month`是一个列表,用于存储每个月份的天数。例如,对于平年,`days_in_month=[31,28,31,30,31,30,31,31,30,31,30,31]`。
4. 最后,输出计算得到的结果,即该日期是这一年的第几天。
需要注意的是,对于闰年,二月份的天数为29天。因此,需要根据闰年判断的规则,确定该年是否是闰年。通常,闰年的判断规则如下:
1. 能被4整除但不能被100整除的年份是闰年,如2004年就是闰年,1900年不是闰年。
2. 能被400整除的年份也是闰年,如2000年是闰年。
相关推荐
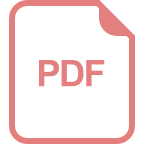
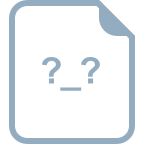












