java list 转map
时间: 2023-08-31 10:32:31 浏览: 160
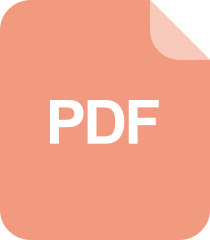
Java lambda list转换map时,把多个参数拼接作为key操作

### 回答1:
你可以使用Java 8中的stream api,它允许你从一个list转换为一个map:list.stream().collect(Collectors.toMap(Function<T, K> keyMapper, Function<T, V> valueMapper));
### 回答2:
Java中可以通过以下两种方法将List转换为Map:
方法一:使用for循环遍历List,将List中的元素作为Map的key,根据需要生成相应的value,并将key和value存入Map中。
示例代码如下:
```java
List<String> list = new ArrayList<>();
list.add("A");
list.add("B");
list.add("C");
Map<String, Integer> map = new HashMap<>();
for (String key : list) {
// 根据需要生成value
int value = ...;
map.put(key, value);
}
```
方法二:使用Java 8的Stream API将List转换为Map。
示例代码如下:
```java
List<String> list = new ArrayList<>();
list.add("A");
list.add("B");
list.add("C");
Map<String, Integer> map = list.stream()
.collect(Collectors.toMap(key -> key, value -> ...));
```
其中,`key -> key`表示将List中的元素作为Map的key,`value -> ...`表示根据需要生成value的逻辑。可以根据实际需求进行修改。
以上是通过两种常用方法将List转换为Map的示例。根据具体情况选择适合的方法进行操作。
### 回答3:
在Java中,我们可以通过以下方法将List转换为Map:
1. 首先,我们需要创建一个空的Map对象,用于存储转换后的数据。
```java
Map<K, V> map = new HashMap<>();
```
这里,K和V分别表示Map的键和值的数据类型。
2. 然后,我们可以使用Java 8的Stream API来转换List为Map。通过Stream的forEach方法遍历List中的每个元素,并使用Lambda表达式将每个元素添加到Map中。
```java
list.stream()
.forEach(e -> map.put(e.getKey(), e.getValue()));
```
在这里,我们假设List中的每个元素都是一个具有getKey和getValue方法的对象,分别用于获取Map中的键和值。
完整的代码示例如下:
```java
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ListToMapExample {
public static void main(String[] args) {
List<Pair> list = ...; // 假设已经有一个List<Pair>对象
Map<Integer, String> map = new HashMap<>();
list.stream()
.forEach(e -> map.put(e.getKey(), e.getValue()));
// 打印转换后的Map
System.out.println(map);
}
}
class Pair {
private int key;
private String value;
public Pair(int key, String value) {
this.key = key;
this.value = value;
}
public int getKey() {
return key;
}
public String getValue() {
return value;
}
}
```
通过以上步骤,我们就可以将List转换为Map了。
阅读全文
相关推荐
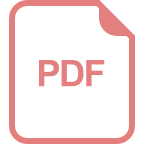
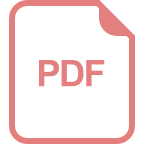






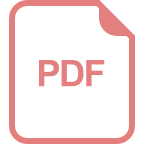
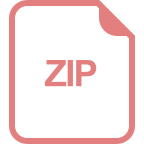
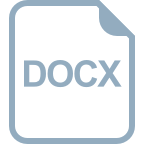
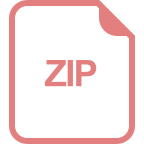
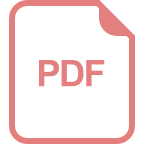
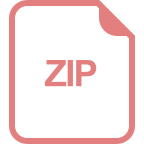