java gui编程写atm机界面并且实现存储,取款等功能
时间: 2024-04-07 11:29:03 浏览: 123
好的,下面是一个Java GUI程序,实现了ATM机界面,并且实现了存储和取款功能:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ATMGUI extends JFrame implements ActionListener{
private JLabel welcomeLabel;
private JLabel idLabel;
private JLabel passwordLabel;
private JTextField idField;
private JPasswordField passwordField;
private JButton loginButton;
private JButton cancelButton;
private JLabel balanceLabel;
private JLabel operationLabel;
private JTextField operationField;
private JButton depositButton;
private JButton withdrawButton;
private double balance;
public ATMGUI() {
init();
this.setTitle("ATM机界面");
this.setSize(400, 300);
this.setLocationRelativeTo(null);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
public void init() {
// 欢迎语
welcomeLabel = new JLabel("欢迎使用ATM机");
welcomeLabel.setFont(new Font("微软雅黑", Font.BOLD, 24));
welcomeLabel.setHorizontalAlignment(SwingConstants.CENTER);
// 用户名和密码输入框
idLabel = new JLabel("账号:");
passwordLabel = new JLabel("密码:");
idField = new JTextField(20);
passwordField = new JPasswordField(20);
// 登录和取消按钮
loginButton = new JButton("登录");
cancelButton = new JButton("取消");
loginButton.addActionListener(this);
cancelButton.addActionListener(this);
// 余额信息
balanceLabel = new JLabel("余额:0.0元");
balanceLabel.setFont(new Font("微软雅黑", Font.BOLD, 16));
balanceLabel.setHorizontalAlignment(SwingConstants.CENTER);
// 存款和取款按钮
operationLabel = new JLabel("请输入操作金额:");
operationField = new JTextField(20);
depositButton = new JButton("存款");
withdrawButton = new JButton("取款");
depositButton.addActionListener(this);
withdrawButton.addActionListener(this);
// 设置布局
this.setLayout(new GridLayout(6, 2, 10, 10));
this.add(welcomeLabel);
this.add(new JLabel(""));
this.add(idLabel);
this.add(idField);
this.add(passwordLabel);
this.add(passwordField);
this.add(loginButton);
this.add(cancelButton);
this.add(balanceLabel);
this.add(new JLabel(""));
this.add(operationLabel);
this.add(operationField);
this.add(depositButton);
this.add(withdrawButton);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == loginButton) {
// 登录
String id = idField.getText();
String password = new String(passwordField.getPassword());
if (id.equals("123456") && password.equals("123456")) {
JOptionPane.showMessageDialog(this, "登录成功", "提示", JOptionPane.INFORMATION_MESSAGE);
balance = 1000.0;
balanceLabel.setText("余额:" + balance + "元");
idField.setEditable(false);
passwordField.setEditable(false);
loginButton.setEnabled(false);
cancelButton.setText("退出");
} else {
JOptionPane.showMessageDialog(this, "账号或密码错误", "错误", JOptionPane.ERROR_MESSAGE);
}
} else if (e.getSource() == cancelButton) {
// 取消或退出
if (cancelButton.getText().equals("取消")) {
idField.setText("");
passwordField.setText("");
} else {
System.exit(0);
}
} else if (e.getSource() == depositButton) {
// 存款
String str = operationField.getText();
if (str.equals("")) {
JOptionPane.showMessageDialog(this, "请输入操作金额", "错误", JOptionPane.ERROR_MESSAGE);
} else {
double amount = Double.parseDouble(str);
balance += amount;
balanceLabel.setText("余额:" + balance + "元");
operationField.setText("");
JOptionPane.showMessageDialog(this, "存款成功", "提示", JOptionPane.INFORMATION_MESSAGE);
}
} else if (e.getSource() == withdrawButton) {
// 取款
String str = operationField.getText();
if (str.equals("")) {
JOptionPane.showMessageDialog(this, "请输入操作金额", "错误", JOptionPane.ERROR_MESSAGE);
} else {
double amount = Double.parseDouble(str);
if (amount > balance) {
JOptionPane.showMessageDialog(this, "余额不足", "错误", JOptionPane.ERROR_MESSAGE);
} else {
balance -= amount;
balanceLabel.setText("余额:" + balance + "元");
operationField.setText("");
JOptionPane.showMessageDialog(this, "取款成功", "提示", JOptionPane.INFORMATION_MESSAGE);
}
}
}
}
public static void main(String[] args) {
new ATMGUI();
}
}
```
这个程序会生成一个ATM机的登录界面,包含一个欢迎语、一个账号输入框、一个密码输入框以及一个登录按钮和一个取消按钮。登录成功后,会显示余额信息以及存款和取款按钮。你可以在代码中进一步完善其他功能。
阅读全文
相关推荐
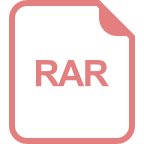
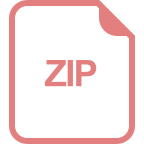
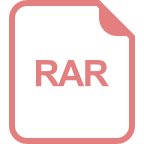
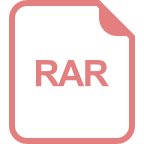
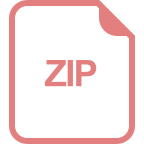
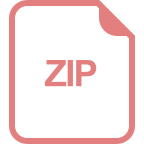
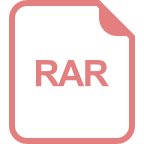
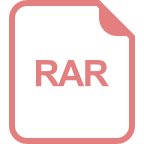
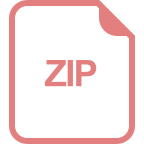
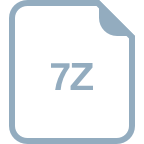
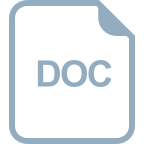
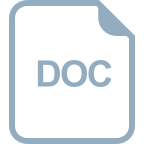
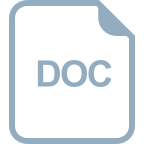
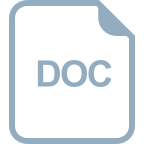
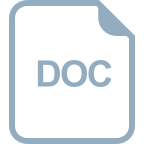
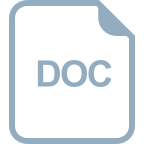