用STM32F4库函数写ADC的DMA2中断函数
时间: 2024-01-01 16:04:52 浏览: 144
以下是使用STM32F4库函数编写ADC DMA2中断函数的示例代码:
```
void DMA2_Stream0_IRQHandler(void)
{
if(DMA_GetITStatus(DMA2_Stream0, DMA_IT_TCIF0))
{
DMA_ClearITPendingBit(DMA2_Stream0, DMA_IT_TCIF0);
// 处理ADC采集到的数据
for(uint16_t i = 0; i < ADC_BUFFER_SIZE; i++)
{
// 获取ADC采集到的数据
uint16_t value = ADC_Buffer[i];
// 在这里进行数据处理或者传输等操作
// ...
// 清空ADC缓存数组
ADC_Buffer[i] = 0;
}
}
}
```
在此示例代码中,我们使用了库函数`DMA_GetITStatus`和`DMA_ClearITPendingBit`来检查和清除DMA中断标志位。在DMA传输完成时,我们可以在中断函数中处理采集到的数据。在这个例子中,我们使用`ADC_Buffer`数组存储采集到的数据。在数据处理完成后,我们需要将数组清空,以便下一次采集。
相关问题
用STM32F4库函数写ADC采样数据通过DMA存储到乒乓buffer,DMA中断切换buffer
以下是使用STM32F4库函数编写的ADC采样数据存储到乒乓buffer并通过DMA切换buffer的示例代码:
首先,需要定义两个大小为缓冲区大小的数组作为乒乓buffer:
```
#define BUFFER_SIZE 1024
volatile uint16_t buffer1[BUFFER_SIZE];
volatile uint16_t buffer2[BUFFER_SIZE];
volatile uint16_t* current_buffer = buffer1; // 当前使用的缓冲区
```
接下来,需要配置ADC和DMA:
```
// 配置ADC
ADC_InitTypeDef ADC_InitStruct;
ADC_CommonInitTypeDef ADC_CommonInitStruct;
DMA_InitTypeDef DMA_InitStruct;
// 配置 ADC
ADC_CommonInitStruct.ADC_Prescaler = ADC_Prescaler_Div4;
ADC_CommonInit(&ADC_CommonInitStruct);
ADC_InitStruct.ADC_Resolution = ADC_Resolution_12b;
ADC_InitStruct.ADC_ScanConvMode = DISABLE;
ADC_InitStruct.ADC_ContinuousConvMode = ENABLE;
ADC_InitStruct.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None;
ADC_InitStruct.ADC_DataAlign = ADC_DataAlign_Right;
ADC_InitStruct.ADC_NbrOfConversion = 1;
ADC_Init(ADC1, &ADC_InitStruct);
ADC_RegularChannelConfig(ADC1, ADC_Channel_0, 1, ADC_SampleTime_3Cycles);
// 配置 DMA
DMA_InitStruct.DMA_Channel = DMA_Channel_0;
DMA_InitStruct.DMA_PeripheralBaseAddr = (uint32_t) &ADC1->DR;
DMA_InitStruct.DMA_Memory0BaseAddr = (uint32_t) buffer1;
DMA_InitStruct.DMA_DIR = DMA_DIR_PeripheralToMemory;
DMA_InitStruct.DMA_BufferSize = BUFFER_SIZE;
DMA_InitStruct.DMA_PeripheralInc = DMA_PeripheralInc_Disable;
DMA_InitStruct.DMA_MemoryInc = DMA_MemoryInc_Enable;
DMA_InitStruct.DMA_PeripheralDataSize = DMA_PeripheralDataSize_HalfWord;
DMA_InitStruct.DMA_MemoryDataSize = DMA_MemoryDataSize_HalfWord;
DMA_InitStruct.DMA_Mode = DMA_Mode_Circular;
DMA_InitStruct.DMA_Priority = DMA_Priority_High;
DMA_InitStruct.DMA_FIFOMode = DMA_FIFOMode_Disable;
DMA_InitStruct.DMA_FIFOThreshold = DMA_FIFOThreshold_HalfFull;
DMA_InitStruct.DMA_MemoryBurst = DMA_MemoryBurst_Single;
DMA_InitStruct.DMA_PeripheralBurst = DMA_PeripheralBurst_Single;
DMA_Init(DMA2_Stream0, &DMA_InitStruct);
DMA_Cmd(DMA2_Stream0, ENABLE);
// 启动 ADC
ADC_Cmd(ADC1, ENABLE);
ADC_SoftwareStartConv(ADC1);
```
其中,ADC使用了PA0作为输入通道,DMA使用了DMA2的stream0通道。注意,这里开启了循环模式,即DMA会一直从ADC采样数据并存储到缓冲区中。
接下来,需要在DMA的中断回调函数中进行缓冲区的切换:
```
void DMA2_Stream0_IRQHandler(void)
{
if (DMA_GetITStatus(DMA2_Stream0, DMA_IT_TCIF0))
{
DMA_ClearITPendingBit(DMA2_Stream0, DMA_IT_TCIF0);
// 切换缓冲区
if (current_buffer == buffer1)
{
current_buffer = buffer2;
DMA2_Stream0->M0AR = (uint32_t) buffer1;
}
else
{
current_buffer = buffer1;
DMA2_Stream0->M0AR = (uint32_t) buffer2;
}
}
}
```
在这里,使用了一个volatile指针current_buffer来指向当前使用的缓冲区。在中断回调函数中,如果检测到了DMA传输完成的中断,就进行缓冲区的切换,并更新DMA的M0AR寄存器指向新的缓冲区地址。
最后,可以使用current_buffer指针来获取当前正在使用的缓冲区中的采样数据:
```
uint16_t sample = current_buffer[index];
```
其中,index是采样数据的索引,可以在0到BUFFER_SIZE-1之间取值。注意,由于current_buffer是一个volatile指针,编译器可能会产生额外的代码来保证读写操作的正确性,因此在频繁读取采样数据时需要注意性能问题。
用STM32F4库函数实现ADC连续采样,DMA双缓冲模式,DMA中断
实现ADC连续采样,DMA双缓冲模式和DMA中断的步骤如下:
1. 配置ADC和DMA
```
// ADC配置
ADC_InitTypeDef ADC_InitStruct;
ADC_CommonInitTypeDef ADC_CommonInitStruct;
ADC_StructInit(&ADC_InitStruct);
ADC_CommonStructInit(&ADC_CommonInitStruct);
ADC_CommonInitStruct.ADC_DMAAccessMode = ADC_DMAAccessMode_2; // DMA双缓冲模式
ADC_CommonInit(&ADC_CommonInitStruct);
ADC_InitStruct.ADC_ContinuousConvMode = ENABLE; // 连续采样模式
ADC_InitStruct.ADC_ExternalTrigConvEdge = ADC_ExternalTrigConvEdge_None; // 外部触发禁用
ADC_InitStruct.ADC_DataAlign = ADC_DataAlign_Right; // 右对齐
ADC_InitStruct.ADC_NbrOfConversion = 1; // 一次转换
ADC_Init(ADC1, &ADC_InitStruct);
// DMA配置
DMA_InitTypeDef DMA_InitStruct;
DMA_StructInit(&DMA_InitStruct);
DMA_InitStruct.DMA_Channel = DMA_Channel_0;
DMA_InitStruct.DMA_PeripheralBaseAddr = (uint32_t)&ADC1->DR;
DMA_InitStruct.DMA_Memory0BaseAddr = (uint32_t)&adc_value[0];
DMA_InitStruct.DMA_DIR = DMA_DIR_PeripheralToMemory;
DMA_InitStruct.DMA_BufferSize = 2; // 双缓冲
DMA_InitStruct.DMA_PeripheralInc = DMA_PeripheralInc_Disable;
DMA_InitStruct.DMA_MemoryInc = DMA_MemoryInc_Enable;
DMA_InitStruct.DMA_PeripheralDataSize = DMA_PeripheralDataSize_HalfWord;
DMA_InitStruct.DMA_MemoryDataSize = DMA_MemoryDataSize_HalfWord;
DMA_InitStruct.DMA_Mode = DMA_Mode_Circular;
DMA_InitStruct.DMA_Priority = DMA_Priority_High;
DMA_InitStruct.DMA_FIFOMode = DMA_FIFOMode_Disable;
DMA_InitStruct.DMA_FIFOThreshold = DMA_FIFOThreshold_HalfFull;
DMA_InitStruct.DMA_MemoryBurst = DMA_MemoryBurst_Single;
DMA_InitStruct.DMA_PeripheralBurst = DMA_PeripheralBurst_Single;
DMA_Init(DMA2_Stream0, &DMA_InitStruct);
DMA_DoubleBufferModeConfig(DMA2_Stream0, (uint32_t)&adc_value[1], DMA_Memory_0);
DMA_DoubleBufferModeCmd(DMA2_Stream0, ENABLE);
```
2. 配置ADC和DMA中断
```
NVIC_InitTypeDef NVIC_InitStruct;
// ADC中断配置
NVIC_InitStruct.NVIC_IRQChannel = ADC_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
// DMA中断配置
NVIC_InitStruct.NVIC_IRQChannel = DMA2_Stream0_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
DMA_ITConfig(DMA2_Stream0, DMA_IT_TC | DMA_IT_HT, ENABLE);
```
3. 启动ADC和DMA
```
ADC_Cmd(ADC1, ENABLE);
DMA_Cmd(DMA2_Stream0, ENABLE);
ADC_DMACmd(ADC1, ENABLE);
```
4. 编写ADC和DMA中断服务函数
```
void ADC_IRQHandler(void)
{
if (ADC_GetITStatus(ADC1, ADC_IT_EOC) == SET)
{
ADC_ClearITPendingBit(ADC1, ADC_IT_EOC);
}
}
void DMA2_Stream0_IRQHandler(void)
{
if (DMA_GetITStatus(DMA2_Stream0, DMA_IT_TCIF0) == SET)
{
DMA_ClearITPendingBit(DMA2_Stream0, DMA_IT_TCIF0);
// 处理DMA传输完成事件
}
else if (DMA_GetITStatus(DMA2_Stream0, DMA_IT_HTIF0) == SET)
{
DMA_ClearITPendingBit(DMA2_Stream0, DMA_IT_HTIF0);
// 处理DMA半传输完成事件
}
}
```
阅读全文
相关推荐



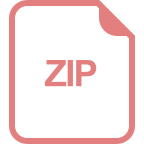
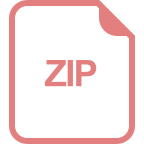
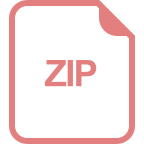

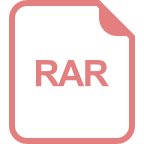
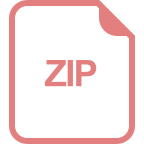
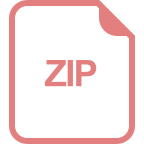
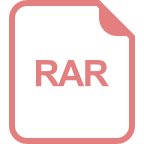

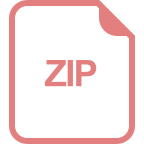
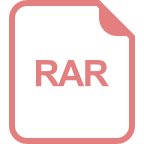