用element-ui 写一个有姓名,密码,手机号码,头像的个人信息的vue页面
时间: 2024-06-10 18:07:24 浏览: 5
好的,这个问题属于技术问题,可以回答。首先你需要安装element-ui依赖,然后在Vue的组件中引入el-form, el-form-item, el-input, el-upload等element-ui的组件来实现个人信息页面的开发。具体实现可以看element-ui的官网中文文档或者相关教程。希望能帮到你。
相关问题
vue协同element-ui带样式的个人信息页面,包含用户名,邮箱,性别,账号,生日,头像字段,头像为圆框
下面是一个使用Vue和Element UI的带样式的个人信息页面示例,包含了用户名、邮箱、性别、账号、生日和头像字段,并将头像显示为圆框:
```html
<template>
<div class="profile">
<h1>个人信息</h1>
<div class="avatar">
<el-avatar :src="user.avatar" :size="120" shape="circle"></el-avatar>
</div>
<div class="info">
<el-row>
<el-col :span="4"><strong>用户名:</strong></el-col>
<el-col :span="20">{{ user.name }}</el-col>
</el-row>
<el-row>
<el-col :span="4"><strong>邮箱:</strong></el-col>
<el-col :span="20">{{ user.email }}</el-col>
</el-row>
<el-row>
<el-col :span="4"><strong>性别:</strong></el-col>
<el-col :span="20">{{ user.gender }}</el-col>
</el-row>
<el-row>
<el-col :span="4"><strong>账号:</strong></el-col>
<el-col :span="20">{{ user.account }}</el-col>
</el-row>
<el-row>
<el-col :span="4"><strong>生日:</strong></el-col>
<el-col :span="20">{{ user.birthday }}</el-col>
</el-row>
</div>
</div>
</template>
<script>
export default {
data() {
return {
user: {
name: 'John Doe',
email: 'john@example.com',
gender: '男',
account: 'johndoe123',
birthday: '1990-01-01',
avatar: 'path/to/avatar.jpg'
}
};
}
};
</script>
<style>
.profile {
display: flex;
flex-direction: column;
align-items: center;
}
.avatar {
margin-top: 20px;
}
.info {
margin-top: 20px;
}
.info el-row {
margin-bottom: 10px;
}
.info strong {
font-weight: bold;
}
</style>
```
在这个示例中,我使用了Element UI的`el-avatar`组件来显示用户的头像,设置了大小为120,形状为圆形。个人信息的字段使用了`el-row`和`el-col`布局,通过设置不同的列宽来实现字段和内容的对齐。你可以根据自己的需求进一步调整样式和布局。
springboot +vue +element-ui实现头像上传
要实现头像上传,可以结合Spring Boot后端框架,Vue前端框架以及Element UI组件库进行实现。
首先,在Vue前端页面中,可以使用Element UI中的Upload组件实现文件上传功能。可以在页面中定义一个Upload组件,设置action属性为上传接口的URL,设置headers属性为请求头部信息,设置on-success属性为上传成功后的回调函数。具体代码如下:
```
<template>
<div>
<el-upload
class="avatar-uploader"
action="/api/uploadAvatar"
:headers="{ Authorization: 'Bearer ' + token }"
:show-file-list="false"
:on-success="handleSuccess">
<img v-if="imageUrl" :src="imageUrl" class="avatar">
<i v-else class="el-icon-plus avatar-uploader-icon"></i>
</el-upload>
</div>
</template>
<script>
import { getToken } from '@/utils/auth'
export default {
data() {
return {
imageUrl: '',
token: getToken()
}
},
methods: {
handleSuccess(response) {
this.imageUrl = response.data.url
}
}
}
</script>
```
其中,token是用于认证的令牌,可以通过getToken函数获取。handleSuccess函数是上传成功后的回调函数,其中response.data.url表示上传成功后的图片URL。
然后,在Spring Boot后端接口中,可以使用Spring MVC的注解@RequestParam来接收上传的文件。具体代码如下:
```
@RestController
@RequestMapping("/api")
public class UploadController {
@PostMapping("/uploadAvatar")
public JsonResult uploadAvatar(@RequestParam("file") MultipartFile file) throws IOException {
// 处理上传的文件
return JsonResult.ok("url", "http://www.example.com/avatar.jpg");
}
}
```
其中,@PostMapping注解表示接收POST请求,@RequestParam("file")注解表示接收名为file的文件参数。处理上传的文件后,可以返回一个JsonResult对象,其中包含上传成功后的图片URL。
相关推荐
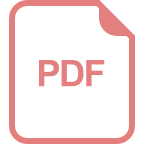
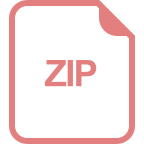
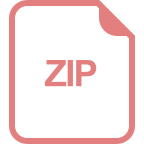












