输入n个朋友的信息,包括姓名、生日、电话号码,本题要求编写程序,按照年龄从大到小的顺序依次输出通讯录。题目保证所有人的生日均不相同。用c语言写
时间: 2024-05-22 12:12:47 浏览: 25
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义结构体
struct friend_info {
char name[20]; // 姓名
char birthday[9]; // 生日
char phone[12]; // 电话号码
int age; // 年龄
};
// 计算年龄
int calculate_age(char* birthday) {
int year = (birthday[0] - '0') * 1000 + (birthday[1] - '0') * 100 + (birthday[2] - '0') * 10 + (birthday[3] - '0');
int month = (birthday[4] - '0') * 10 + (birthday[5] - '0');
int day = (birthday[6] - '0') * 10 + (birthday[7] - '0');
time_t t = time(NULL);
struct tm tm = *localtime(&t);
int age = tm.tm_year + 1900 - year;
if (tm.tm_mon + 1 < month || (tm.tm_mon + 1 == month && tm.tm_mday < day)) {
age--;
}
return age;
}
// 比较函数,按照年龄从大到小排序
int cmp(const void* a, const void* b) {
struct friend_info* friend_a = (struct friend_info*)a;
struct friend_info* friend_b = (struct friend_info*)b;
return friend_b->age - friend_a->age;
}
int main() {
int n;
scanf("%d", &n);
struct friend_info* friends = (struct friend_info*)malloc(sizeof(struct friend_info) * n);
// 输入信息并计算年龄
for (int i = 0; i < n; i++) {
scanf("%s %s %s", friends[i].name, friends[i].birthday, friends[i].phone);
friends[i].age = calculate_age(friends[i].birthday);
}
// 按照年龄排序
qsort(friends, n, sizeof(struct friend_info), cmp);
// 输出通讯录
for (int i = 0; i < n; i++) {
printf("%s %s %s\n", friends[i].name, friends[i].birthday, friends[i].phone);
}
free(friends);
return 0;
}
相关推荐
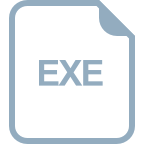
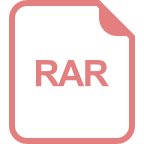






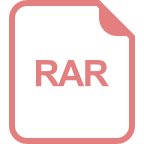
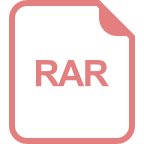
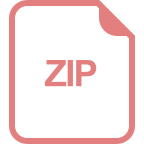
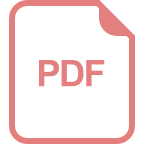