Python写加密算法并给出解密算法
时间: 2023-03-19 11:23:39 浏览: 155
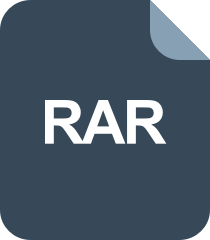
加密解密算法

Python可以使用多种加密算法,例如对称加密算法和非对称加密算法。以下是一个简单的对称加密算法的Python示例代码:
```python
import base64
from cryptography.fernet import Fernet
# 生成密钥
key = Fernet.generate_key()
fernet = Fernet(key)
# 要加密的明文
message = b"Hello world"
# 加密
encrypted_message = fernet.encrypt(message)
# 将密钥和加密后的消息进行base64编码并输出
print(base64.urlsafe_b64encode(key).decode())
print(base64.urlsafe_b64encode(encrypted_message).decode())
# 解密
decrypted_message = fernet.decrypt(encrypted_message)
# 输出解密后的明文
print(decrypted_message.decode())
```
该代码生成一个随机密钥并使用Fernet算法进行加密和解密。可以将密钥和加密后的消息进行base64编码后输出,以便在不同的系统之间传输。要解密消息,只需使用相同的密钥和Fernet对象调用decrypt()方法即可。
需要注意的是,对称加密算法中使用的密钥必须安全地存储和传输,否则可能会被攻击者获取并使用。
阅读全文
相关推荐
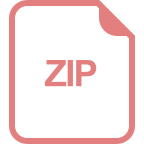
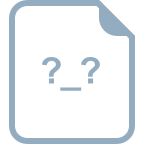
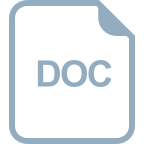












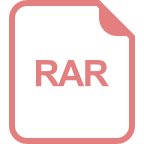