cmake里面引入动态库 并使用的例子
时间: 2023-07-21 07:12:39 浏览: 46
假设有一个名为`myapp`的可执行文件,需要引入名为`libfoo.so`的动态库并使用其中的函数,可以按照以下步骤进行:
1. 创建`CMakeLists.txt`文件,并在其中添加以下内容:
```
cmake_minimum_required(VERSION 3.5)
project(myapp)
add_executable(myapp main.cpp)
find_library(foo NAMES foo PATHS /usr/local/lib)
target_link_libraries(myapp ${foo})
```
其中,`main.cpp`是`myapp`的源文件,`foo`是要引入的动态库的名称,`/usr/local/lib`是`libfoo.so`所在的路径。
2. 创建`main.cpp`文件,并在其中使用`libfoo.so`中的函数:
```c++
#include <iostream>
#include <dlfcn.h>
typedef int (*foo_func_t)(int, int);
int main()
{
void *handle = dlopen("libfoo.so", RTLD_LAZY);
if (!handle) {
std::cerr << "Error: " << dlerror() << std::endl;
return 1;
}
foo_func_t foo_func = (foo_func_t)dlsym(handle, "foo");
if (!foo_func) {
std::cerr << "Error: " << dlerror() << std::endl;
dlclose(handle);
return 1;
}
int result = foo_func(3, 4);
std::cout << "Result: " << result << std::endl;
dlclose(handle);
return 0;
}
```
在`main.cpp`中,首先使用`dlopen`函数打开`libfoo.so`动态库,然后使用`dlsym`函数获取其中的`foo`函数,并将其保存到函数指针变量`foo_func`中。最后,调用`foo_func`函数并输出结果。
注意,这里使用了`dlfcn.h`头文件中的函数,需要在编译时链接`dl`库,可以在`CMakeLists.txt`中添加以下命令:
```
target_link_libraries(myapp dl)
```
3. 在命令行中执行以下命令进行编译:
```
mkdir build
cd build
cmake ..
make
```
编译完成后,可以运行`myapp`可执行文件,输出结果应为`Result: 7`。
相关推荐
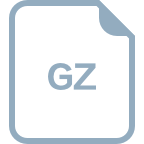
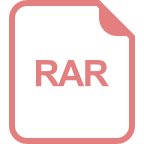
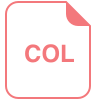
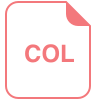












