编写代码,在windows下用C++解压zip文件并无条件替换目标目录下的原有文件
时间: 2024-04-29 16:27:12 浏览: 28
你好,关于你的问题,我可以提供以下代码段给你参考:
```
#include <windows.h>
#include <tchar.h>
#include <stdio.h>
#include <zip.h>
int UnzipFile(LPCTSTR lpszFilePath, LPCTSTR lpszDir)
{
int nRet = 0;
unzFile hZip = unzOpen(lpszFilePath);
if (!hZip)
{
nRet = -1;
return nRet;
}
unz_global_info globalInfo = { 0 };
if (unzGetGlobalInfo(hZip, &globalInfo) != UNZ_OK)
{
nRet = -2;
unzClose(hZip);
return nRet;
}
char szPath[MAX_PATH] = { 0 };
if (unzGoToFirstFile(hZip) != UNZ_OK)
{
nRet = -3;
unzClose(hZip);
return nRet;
}
do
{
unz_file_info fileInfo = { 0 };
char szFileName[MAX_PATH] = { 0 };
if (unzGetCurrentFileInfo(hZip, &fileInfo, szFileName, MAX_PATH, NULL, 0, NULL, 0) != UNZ_OK)
{
nRet = -4;
unzClose(hZip);
return nRet;
}
_snprintf_s(szPath, MAX_PATH, "%s%s", lpszDir, szFileName);
DWORD dwAttrs = GetFileAttributesA(szPath);
if (dwAttrs != INVALID_FILE_ATTRIBUTES)
{
if ((dwAttrs & FILE_ATTRIBUTE_DIRECTORY) == FILE_ATTRIBUTE_DIRECTORY)
{
if (!RemoveDirectoryA(szPath))
{
nRet = -5;
unzClose(hZip);
return nRet;
}
}
else
{
if (!DeleteFileA(szPath))
{
nRet = -6;
unzClose(hZip);
return nRet;
}
}
}
if (unzOpenCurrentFile(hZip) != UNZ_OK)
{
nRet = -7;
unzClose(hZip);
return nRet;
}
FILE* fp = NULL;
fopen_s(&fp, szPath, "wb");
if (!fp)
{
nRet = -8;
unzCloseCurrentFile(hZip);
unzClose(hZip);
return nRet;
}
char* pBuffer = new char[fileInfo.uncompressed_size];
if (!pBuffer)
{
nRet = -9;
fclose(fp);
unzCloseCurrentFile(hZip);
unzClose(hZip);
return nRet;
}
if (unzReadCurrentFile(hZip, pBuffer, fileSize) < 0)
{
nRet = -10;
delete[] pBuffer;
fclose(fp);
unzCloseCurrentFile(hZip);
unzClose(hZip);
return nRet;
}
fwrite(pBuffer, fileInfo.uncompressed_size, 1, fp);
delete[] pBuffer;
fclose(fp);
unzCloseCurrentFile(hZip);
} while (unzGoToNextFile(hZip) == UNZ_OK);
unzClose(hZip);
return nRet;
}
int main()
{
LPCTSTR lpszFilePath = _T("D:\\file.zip");
LPCTSTR lpszDir = _T("D:\\TargetPath\\");
return UnzipFile(lpszFilePath, lpszDir);
}
```
这是一个在Windows下用C语言解压zip文件并替换目标目录下原文件的方法。你可以根据自己的需求适当修改代码。如果你有其他问题,可以随时问我。
阅读全文
相关推荐
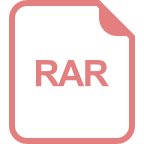
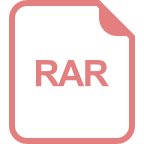
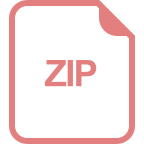
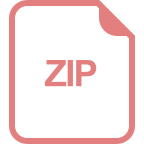
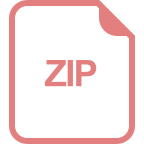
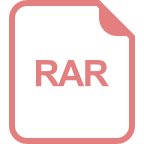
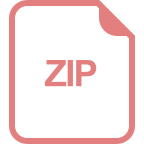
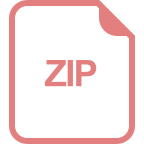
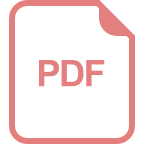
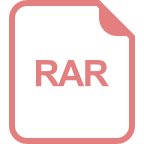
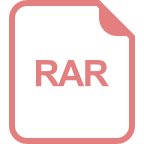
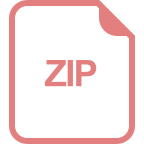
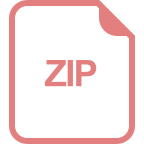
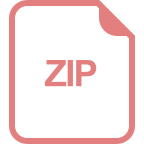
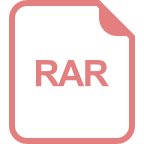