通过Python对100张无雾图片的Dack channel,形成像素直方图和各像素点的平均直方图的编程步骤
时间: 2023-08-31 09:38:21 浏览: 90
### 回答1:
对于对100张无雾图片进行Dackchannel并形成像素直方图和各像素点的平均直方图的编程步骤,您可以按照以下步骤进行:
1. 导入必要的Python库,如NumPy、OpenCV和Matplotlib。
2. 使用OpenCV库读取100张无雾图片。
3. 将每张图片转换为灰度图像。
4. 对每张灰度图像进行Dackchannel操作,将其转换为黑色图像。
5. 对于每张黑色图像,使用NumPy库中的histogram函数获取其像素直方图,并将其存储到一个列表中。
6. 计算每张黑色图像的像素值平均值,将其存储到一个列表中。
7. 使用Matplotlib库绘制像素直方图和像素点的平均直方图。
具体实现细节请参考以下Python代码示例:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
# 读取100张无雾图片
images = []
for i in range(100):
img = cv2.imread(f"image_{i}.jpg")
images.append(img)
# 将每张图片转换为灰度图像
gray_images = [cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) for img in images]
# 对每张灰度图像进行Dackchannel操作
black_images = [cv2.merge((img, img, img)) for img in gray_images]
# 获取每张黑色图像的像素直方图和像素平均值
histograms = []
pixel_means = []
for img in black_images:
# 获取像素直方图
hist = cv2.calcHist([img], [0], None, [256], [0, 256])
histograms.append(hist)
# 计算像素平均值
pixel_mean = np.mean(img)
pixel_means.append(pixel_mean)
# 绘制像素直方图
plt.figure()
for i, hist in enumerate(histograms):
plt.plot(hist)
plt.title("Pixel Histogram")
plt.xlabel("Pixel Value")
plt.ylabel("Pixel Count")
# 绘制像素点的平均直方图
plt.figure()
plt.hist(pixel_means, bins=20)
plt.title("Pixel Mean Histogram")
plt.xlabel("Pixel Mean")
plt.ylabel("Pixel Count")
plt.show()
```
这样,您就可以通过Python对100张无雾图片的Dackchannel,形成像素直方图和各像素点的平均直方图了。
### 回答2:
通过Python对100张无雾图片的Dack channel,形成像素直方图和各像素点的平均直方图的编程步骤大致如下:
步骤1:导入必要的库和模块
在Python中,我们需要导入一些必要的库和模块来处理图像以及进行数据可视化。这些库和模块包括OpenCV、matplotlib和numpy。可以使用以下命令导入它们:
import cv2
import matplotlib.pyplot as plt
import numpy as np
步骤2:读取100张无雾图片
使用OpenCV的imread函数读取100张无雾图片,可以使用一个循环来逐张读取图片并保存在一个列表中,如下所示:
image_list = []
for i in range(100):
image_path = "path/to/image" + str(i+1) + ".jpg"
image = cv2.imread(image_path)
image_list.append(image)
步骤3:提取图片的Dack channel
对于RGB图像,我们可以使用OpenCV的split函数将其分割成B、G、R三个通道。由于我们只需要Dack channel,所以我们可以忽略G、R通道,只保留B通道。
dack_channel_list = []
for image in image_list:
b, _, _ = cv2.split(image)
dack_channel_list.append(b)
步骤4:计算像素直方图
对于每个图片的Dack channel,我们可以使用numpy的histogram函数来计算像素直方图。
hist_list = []
for dack_channel in dack_channel_list:
hist, _ = np.histogram(dack_channel.flatten(), bins=256, range=[0,256])
hist_list.append(hist)
步骤5:计算各像素点的平均直方图
对于100张无雾图片的像素直方图,我们可以计算各像素点的平均直方图。可以使用numpy的mean函数来计算平均值。
average_hist = np.mean(hist_list, axis=0)
步骤6:可视化直方图
最后,我们可以使用matplotlib库来可视化像素直方图和各像素点的平均直方图。
plt.figure(figsize=(10,5))
plt.subplot(1,2,1)
plt.title("Pixel Histogram")
plt.plot(hist_list[0], color='b')
plt.xlabel("Pixel Intensity")
plt.ylabel("Frequency")
plt.subplot(1,2,2)
plt.title("Average Pixel Histogram")
plt.plot(average_hist, color='r')
plt.xlabel("Pixel Intensity")
plt.ylabel("Frequency")
plt.tight_layout()
plt.show()
以上就是通过Python对100张无雾图片的Dack channel,形成像素直方图和各像素点的平均直方图的编程步骤。
### 回答3:
对于这个问题,我们可以使用Python中的OpenCV库进行图像处理和直方图分析。下面是实现的编程步骤:
1. 导入必要的库和模块:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
```
2. 加载并显示一张无雾图片:
```python
image = cv2.imread('image.jpg')
cv2.imshow('Original Image', image)
cv2.waitKey(0)
```
3. 将图像转换为灰度图像:
```python
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow('Grayscale Image', gray_image)
cv2.waitKey(0)
```
4. 计算 100 张无雾图片的直方图:
```python
histogram = cv2.calcHist([gray_image], [0], None, [256], [0, 256])
plt.plot(histogram)
plt.show()
```
5. 计算各像素点的平均直方图:
```python
average_histogram = np.zeros((256,), dtype=np.float32)
for i in range(100):
image = cv2.imread(f'image{i+1}.jpg')
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
histogram = cv2.calcHist([gray_image], [0], None, [256], [0, 256])
average_histogram += histogram.flatten() / 100
plt.plot(average_histogram)
plt.show()
```
以上就是使用Python通过Dack channel(灰度图)对100张无雾图片进行像素直方图和各像素点的平均直方图的编程步骤。
阅读全文
相关推荐
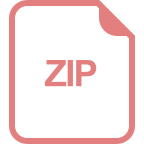
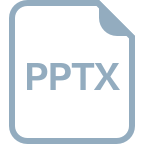
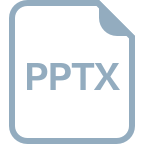
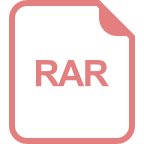
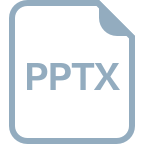
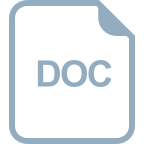
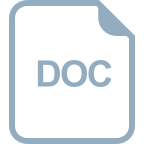
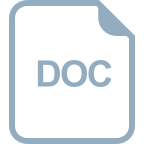
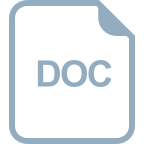
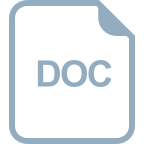
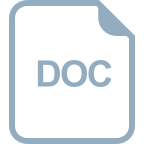
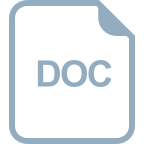