通过python实现对彩色图像的频域模糊及复原
时间: 2023-06-08 20:09:16 浏览: 135
可以通过将彩色图像转换为RGB三个通道的灰度图像,然后分别进行频域模糊和复原。
1. 首先,使用OpenCV库将彩色图像读入,并将其转换为灰度图像:
```python
import cv2
img = cv2.imread('color_image.jpg')
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 然后,使用numpy库中的fft2函数将灰度图像转换为频域图像,并进行频域滤波,例如低通滤波器:
```python
import numpy as np
# 2D fast Fourier transform to convert image to frequency domain
f = np.fft.fft2(gray_img)
# Shift the zero-frequency component to the center of the spectrum
fshift = np.fft.fftshift(f)
# Create a low pass filter to remove high frequency components
rows, cols = gray_img.shape
crow, ccol = rows//2, cols//2
mask = np.zeros((rows, cols), np.uint8)
mask[crow-30:crow+30, ccol-30:ccol+30] = 1
# Apply the mask to the frequency domain image
fshift = fshift * mask
# Shift the zero-frequency component back to the corners of the spectrum
f_ishift = np.fft.ifftshift(fshift)
# 2D inverse fast Fourier transform to convert frequency domain image to spatial image
img_back = np.fft.ifft2(f_ishift)
# Convert the real part of the complex image to an 8-bit grayscale image
img_back = np.abs(img_back).astype(np.uint8)
```
3. 最后,显示频域模糊和复原图像:
```python
# Show the original image, frequency domain blurred image, and recovered image
cv2.imshow('Original Image', img)
cv2.imshow('Frequency Domain Blurred Image', img_back)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样就可以通过Python实现对彩色图像的频域模糊及复原了。
阅读全文
相关推荐
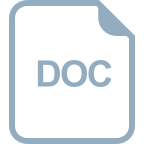
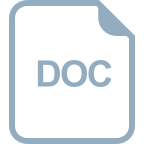
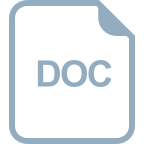

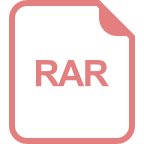
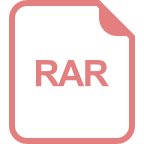
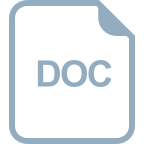
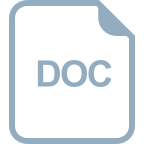
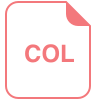
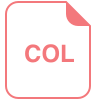
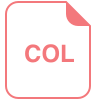
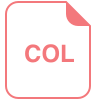
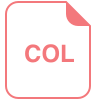
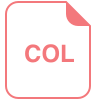
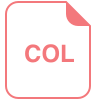
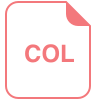
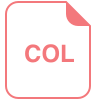
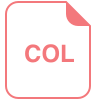
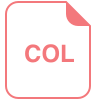