分别尝试采用顺序表和单链表两种不同的存储结构实现由键盘输入学生信息(包括学号和成绩),学号为0作为结束标志。对建成的线性表,再分别采用正确的程序控制方法,输出线性表里储存的信息。
时间: 2023-05-30 22:04:11 浏览: 85
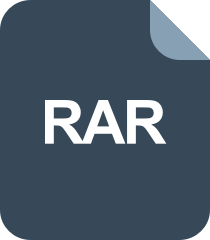
定义一个包含学生信息(学号,姓名,成绩)的顺序表
顺序表实现:
```c++
#include <iostream>
using namespace std;
const int MAX_SIZE = 100; // 线性表的最大长度
struct Student {
int id;
int score;
};
struct SeqList {
Student data[MAX_SIZE];
int length;
};
void init(SeqList& L) {
L.length = 0;
}
void insert(SeqList& L, Student s) {
if (L.length >= MAX_SIZE) {
cout << "Error: List is full!" << endl;
return;
}
L.data[L.length] = s;
L.length++;
}
void print(SeqList L) {
for (int i = 0; i < L.length; i++) {
cout << "Student " << i+1 << ": " << L.data[i].id << " " << L.data[i].score << endl;
}
}
int main() {
SeqList L;
init(L);
Student s;
while (true) {
cout << "Enter student id and score (id=0 to end): ";
cin >> s.id;
if (s.id == 0) {
break;
}
cin >> s.score;
insert(L, s);
}
print(L);
return 0;
}
```
单链表实现:
```c++
#include <iostream>
using namespace std;
struct Student {
int id;
int score;
Student* next;
};
void insert(Student*& head, Student* s) {
if (head == nullptr) {
head = s;
return;
}
Student* p = head;
while (p->next != nullptr) {
p = p->next;
}
p->next = s;
}
void print(Student* head) {
int i = 1;
Student* p = head;
while (p != nullptr) {
cout << "Student " << i++ << ": " << p->id << " " << p->score << endl;
p = p->next;
}
}
int main() {
Student* head = nullptr;
Student* s;
while (true) {
cout << "Enter student id and score (id=0 to end): ";
s = new Student;
cin >> s->id;
if (s->id == 0) {
delete s;
break;
}
cin >> s->score;
s->next = nullptr;
insert(head, s);
}
print(head);
return 0;
}
```
阅读全文
相关推荐
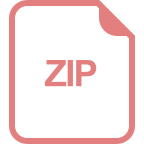
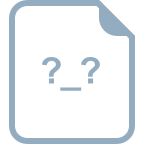
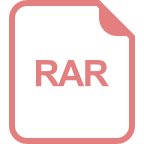
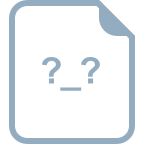
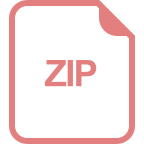
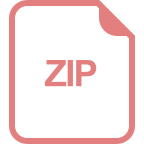
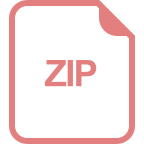
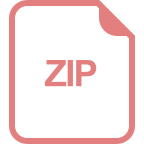
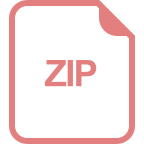
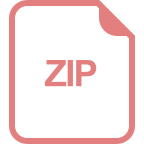