编写一个应用程序来创建两个线程。一个线程打印1-100之间的所有奇数,另一个线程打印1-100之间的所有偶数。这两个线程需要随机睡眠一段时间,然后继续打印下一个数字。使用Runnable接口和Thread类实现编程。
时间: 2023-06-08 19:04:25 浏览: 84
好的,这是一个技术问题,很高兴为你解答。
以下是实现该应用程序的代码:
```java
/**
* 打印数字类
*/
class PrintNumber implements Runnable {
private final int start;
private final int end;
private final boolean odd;
public PrintNumber(int start, int end, boolean odd) {
this.start = start;
this.end = end;
this.odd = odd;
}
@Override
public void run() {
for (int i = start; i <= end; i++) {
if ((i % 2 == 0 && !odd) || (i % 2 == 1 && odd)) {
System.out.println(i);
}
try {
Thread.sleep((int) (Math.random() * 1000));
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) {
Thread oddThread = new Thread(new PrintNumber(1, 100, true));
Thread evenThread = new Thread(new PrintNumber(1, 100, false));
oddThread.start();
evenThread.start();
}
}
```
以上代码使用了Runnable接口和Thread类来实现两个线程同时打印1-100之间的奇数和偶数,每个数字输出之前都会随机睡眠一段时间,通过这种方式模拟两个线程并发执行的情况。
相关推荐
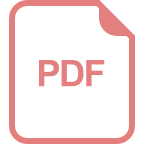
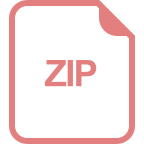














