用opencv写一个qfp封装检测算法
时间: 2023-08-04 17:39:12 浏览: 144
首先,需要安装OpenCV库,并且了解QFP封装的特征。QFP封装是一种常见的芯片封装形式,具有四个直角和四个圆角,是一个矩形形状。
以下是一种检测QFP封装的简单方法:
1. 读取图像并将其转换为灰度图像。
```c++
cv::Mat image = cv::imread("image_path", cv::IMREAD_GRAYSCALE);
```
2. 对图像进行高斯滤波以去除噪声。
```c++
cv::GaussianBlur(image, image, cv::Size(5, 5), 0);
```
3. 对图像进行二值化处理。
```c++
cv::threshold(image, image, 0, 255, cv::THRESH_BINARY | cv::THRESH_OTSU);
```
4. 进行轮廓检测。
```c++
std::vector<std::vector<cv::Point>> contours;
cv::findContours(image, contours, cv::RETR_LIST, cv::CHAIN_APPROX_SIMPLE);
```
5. 遍历所有轮廓并筛选出符合QFP封装形状的轮廓。
```c++
std::vector<cv::Rect> qfp_rects;
for (const auto& contour : contours) {
cv::Rect rect = cv::boundingRect(contour);
// 判断是否符合QFP封装的特征
if (rect.width > rect.height && rect.width / rect.height > 1.2 && rect.width / rect.height < 2.5) {
qfp_rects.push_back(rect);
}
}
```
6. 在原图中绘制符合QFP封装形状的轮廓矩形框。
```c++
for (const auto& rect : qfp_rects) {
cv::rectangle(image, rect, cv::Scalar(0, 0, 255), 2);
}
```
完整代码如下:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image_path", cv::IMREAD_GRAYSCALE);
if (image.empty()) {
std::cout << "Can not open image" << std::endl;
return -1;
}
// 高斯滤波
cv::GaussianBlur(image, image, cv::Size(5, 5), 0);
// 二值化
cv::threshold(image, image, 0, 255, cv::THRESH_BINARY | cv::THRESH_OTSU);
// 轮廓检测
std::vector<std::vector<cv::Point>> contours;
cv::findContours(image, contours, cv::RETR_LIST, cv::CHAIN_APPROX_SIMPLE);
// 筛选QFP封装轮廓
std::vector<cv::Rect> qfp_rects;
for (const auto& contour : contours) {
cv::Rect rect = cv::boundingRect(contour);
// 判断是否符合QFP封装的特征
if (rect.width > rect.height && rect.width / rect.height > 1.2 && rect.width / rect.height < 2.5) {
qfp_rects.push_back(rect);
}
}
// 绘制矩形框
for (const auto& rect : qfp_rects) {
cv::rectangle(image, rect, cv::Scalar(0, 0, 255), 2);
}
cv::imshow("QFP Detection", image);
cv::waitKey(0);
return 0;
}
```
需要注意的是,该方法只是一个简单的QFP封装检测算法,对于一些特殊情况可能无法检测到正确的结果,可以根据实际情况进行调整和改进。
阅读全文
相关推荐
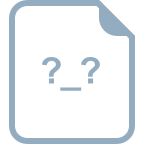
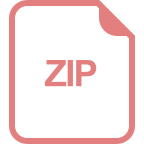
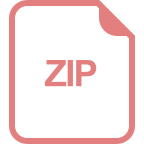
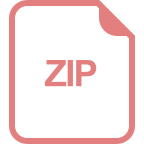
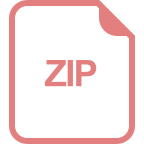
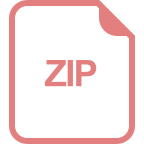
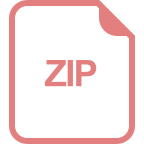
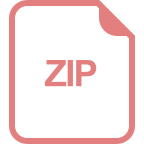
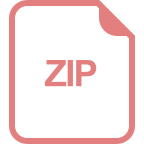
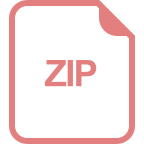
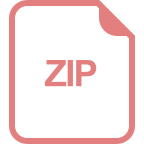
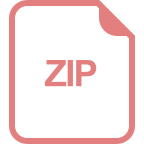
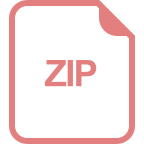