如何实现一个 Starter
时间: 2024-03-21 11:39:27 浏览: 56
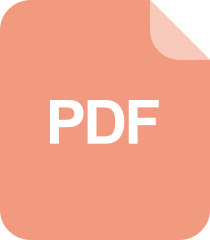
SpringBoot如何实现starter原理详解
在 Spring Boot 中,Starter 是一种特殊的依赖,它能够帮助我们快速地集成一些常用的功能,例如日志、数据库等。如果我们想要实现一个自己的 Starter,可以按照以下步骤来进行:
1. 创建一个 Maven 项目,项目的命名规则为 `spring-boot-starter-{name}`,其中 `{name}` 表示 Starter 的名称,例如 `spring-boot-starter-mybatis`。
2. 在项目的 `pom.xml` 文件中,添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>${spring.boot.version}</version>
<scope>compile</scope>
</dependency>
```
这个依赖表示我们的 Starter 依赖于 Spring Boot 的核心 Starter。
3. 创建一个自定义的 Starter 类,该类需要继承自 `org.springframework.boot.autoconfigure.EnableAutoConfiguration` 类,并且需要添加 `@Configuration` 和 `@ConditionalOnClass` 注解,例如:
```java
@Configuration
@ConditionalOnClass(MyService.class)
@EnableConfigurationProperties(MyProperties.class)
public class MyAutoConfiguration {
@Autowired
private MyProperties properties;
@Bean
@ConditionalOnMissingBean
public MyService myService() {
return new MyService(properties.getName());
}
}
```
上述代码表示,当 MyService 类存在时,才会加载这个 Starter。另外,`@EnableConfigurationProperties(MyProperties.class)` 注解表示需要将 `MyProperties` 类注入到配置文件中。
4. 创建一个自定义的 Properties 类,用于读取配置文件中的属性,例如:
```java
@ConfigurationProperties(prefix = "my")
public class MyProperties {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
```
上述代码中,`@ConfigurationProperties(prefix = "my")` 注解表示该类需要读取配置文件中以 `my` 开头的属性,例如 `my.name`。
5. 将项目打包成 Jar 文件,并上传到 Maven 仓库中。
6. 在其他项目中,可以通过添加以下依赖来使用我们的 Starter:
```xml
<dependency>
<groupId>com.example</groupId>
<artifactId>spring-boot-starter-mybatis</artifactId>
<version>1.0.0</version>
</dependency>
```
上述代码中,`com.example` 表示我们自己的组织名称,`spring-boot-starter-mybatis` 表示我们自己的 Starter 名称,`1.0.0` 表示版本号。
通过以上步骤,我们就成功地实现了一个自己的 Starter,可以方便地在其他项目中使用。
阅读全文
相关推荐
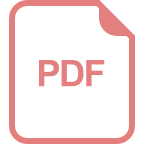
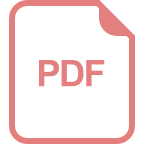
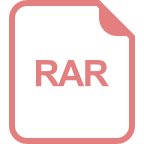
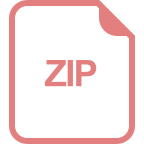
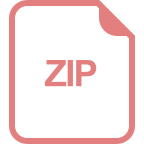
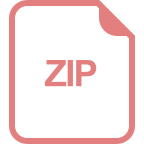
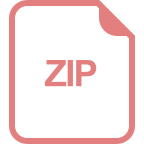
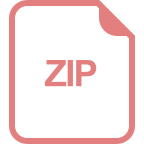
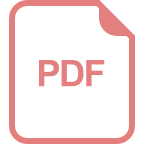
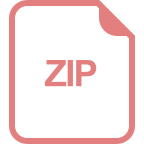
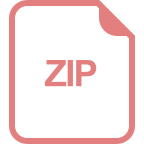
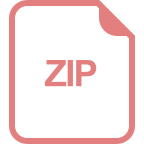
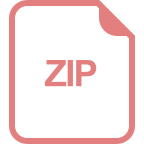
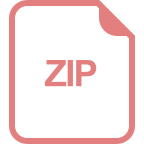
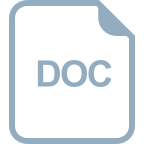
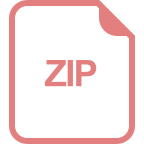