struct chexinxi *duqu() { struct chexinxi *head2=NULL; struct chexinxi *p,*pr; char chexinghao[20], chepaihao[20]; FILE *fp = fopen("车辆信息.txt", "r"); if(fp==NULL) { printf("\t\t\t\t打开文件失败!\n"); } else { while (fscanf(fp, "%s %s", chexinghao, chepaihao) != EOF) { p=(struct chexinxi*)malloc(sizeof(struct chexinxi)); strcpy(p->chexinghao, chexinghao); strcpy(p->chepaihao, chepaihao); if (head2 == NULL) { pr=head2 = p; } else { pr->next=p; pr=p; } } fclose(fp); pr->next=NULL; } return head2; }优化
时间: 2024-01-22 22:02:18 浏览: 26
下面是对这段代码的优化:
1. 添加错误处理。在打开文件失败时,应该输出更多的错误信息,例如文件名和错误码等,方便用户进行问题定位。
2. 简化链表构建过程。可以使用一个临时指针变量 temp,将其始终指向链表的尾节点,将新节点插入到链表的尾部,可以避免大量的 if/else 语句。
3. 检查 malloc 分配内存是否成功。如果分配内存失败,应该进行相应的错误处理。
优化后的代码如下所示:
```c
struct chexinxi *duqu() {
struct chexinxi *head = NULL;
struct chexinxi *temp = NULL;
char chexinghao[20], chepaihao[20];
FILE *fp = fopen("车辆信息.txt", "r");
if (fp == NULL) {
printf("打开文件失败!文件名:%s,错误码:%d\n", "车辆信息.txt", errno);
} else {
while (fscanf(fp, "%s %s", chexinghao, chepaihao) != EOF) {
struct chexinxi *p = malloc(sizeof(struct chexinxi));
if (p == NULL) {
printf("分配内存失败!\n");
exit(1);
}
strcpy(p->chexinghao, chexinghao);
strcpy(p->chepaihao, chepaihao);
if (head == NULL) {
head = temp = p;
} else {
temp->next = p;
temp = p;
}
}
fclose(fp);
if (temp != NULL) {
temp->next = NULL;
}
}
return head;
}
```
通过以上优化,代码的可读性和健壮性都得到了提高。
相关推荐









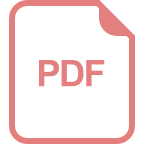
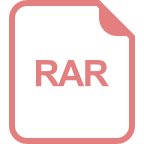
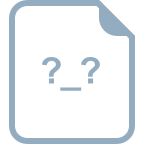