用C++代码写一个自动签到的程序
时间: 2023-09-04 22:04:26 浏览: 229
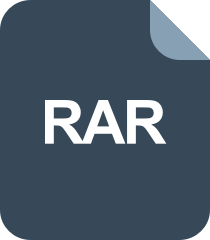
一个简单的C++抽签程序

### 回答1:
以下是一个简单的 C 语言程序示例,实现自动签到功能:
```c
#include <stdio.h>
#include <time.h>
int main()
{
time_t current_time;
time(¤t_time); // 获取当前时间
printf("今天是:%s", ctime(¤t_time)); // 打印当前时间
printf("签到成功!\n");
return 0;
}
```
这段代码通过 `time` 函数获取当前时间,并使用 `ctime` 函数将时间转换成可读格式,然后使用 `printf` 函数输出。最后程序会输出 "签到成功!"。
需要注意的是,这个程序只是简单的演示了如何使用 C 语言获取当前时间并输出,实际应用中需要根据具体情况来更改程序。
希望对您有所帮助。
### 回答2:
自动签到程序可以使用C语言编写,下面是一个简单的示例:
```c
#include <stdio.h>
#include <time.h>
int main() {
// 获取当前时间
time_t now = time(NULL);
struct tm *current_time = localtime(&now);
// 获取当前日期和时间
char date[11];
sprintf(date, "%d-%02d-%02d", current_time->tm_year + 1900, current_time->tm_mon + 1, current_time->tm_mday);
char time[9];
sprintf(time, "%02d:%02d:%02d", current_time->tm_hour, current_time->tm_min, current_time->tm_sec);
// 打印签到信息
printf("自动签到成功!\n");
printf("日期:%s\n", date);
printf("时间:%s\n", time);
return 0;
}
```
这个程序使用`time`函数获取当前时间,然后使用`localtime`函数将时间转换为`struct tm`结构体表示。接着,通过`sprintf`函数将日期和时间格式化为字符串。最后,使用`printf`函数打印签到信息。
注意,以上示例只是一个简单的示例,具体的自动签到逻辑和操作需要根据实际需求来编写。
### 回答3:
自动签到程序可以通过使用C语言编写,实现自动打开网页并进行签到的功能。下面给出一个简单的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <windows.h>
int main() {
ShellExecute(NULL, "open", "http://example.com/signin", NULL, NULL, SW_SHOWDEFAULT); // 调用系统默认的浏览器打开签到页面
Sleep(2000); // 等待2秒,确保页面加载完毕
// 具体填写用户名和密码的代码,请根据实际情况修改
char username[] = "your_username";
char password[] = "your_password";
// 构造执行JavaScript脚本的命令,实现自动填写表单
char command[200];
sprintf(command, "document.getElementById('username').value = '%s';"
"document.getElementById('password').value = '%s';"
"document.getElementById('submit').click();",
username, password);
ShellExecute(NULL, "open", "javascript:", command, NULL, SW_HIDE); // 执行JavaScript脚本实现自动填写表单和提交
return 0;
}
```
上述代码通过使用Windows的ShellExecute函数实现打开指定网页的功能,再通过执行JavaScript脚本实现自动填写表单和提交。需要注意的是,你需要根据实际的网页结构和需要填写的表单元素的id进行相应的修改。
阅读全文
相关推荐
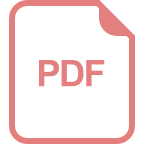

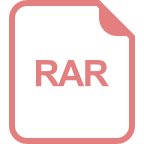
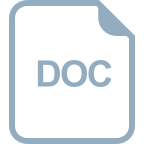
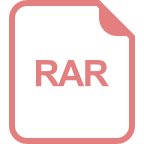
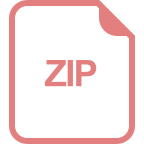
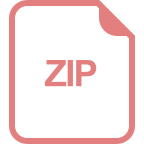
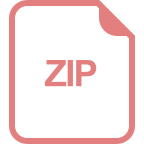
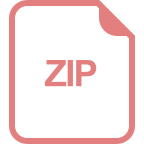
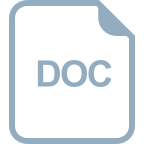
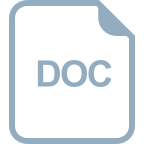
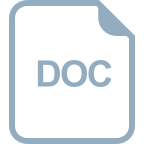
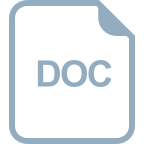
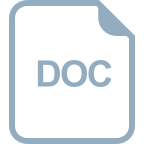
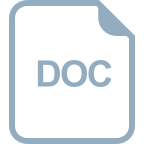
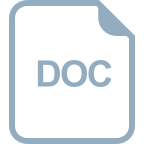
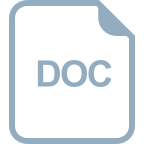