请帮我用 Python 的 Tkinter 模块来创建一个窗口,窗口有两个查询标签,有提交按钮
时间: 2023-04-05 16:01:41 浏览: 174
好的,我可以回答这个问题。以下是使用 Python 的 Tkinter 模块创建一个窗口,窗口有两个查询标签,有提交按钮的代码示例:
```python
import tkinter as tk
# 创建窗口
window = tk.Tk()
# 设置窗口标题
window.title("查询窗口")
# 设置窗口大小
window.geometry("300x200")
# 创建查询标签1
label1 = tk.Label(window, text="查询1")
label1.pack()
# 创建查询标签2
label2 = tk.Label(window, text="查询2")
label2.pack()
# 创建提交按钮
button = tk.Button(window, text="提交")
button.pack()
# 进入消息循环
window.mainloop()
```
希望这个代码示例能够帮助你创建一个带有查询标签和提交按钮的窗口。
相关问题
python使用Tkinter创建一个窗口自适应,有两列文本框,中间分布有三个按钮的界面
### 回答1:
可以使用 Tkinter 库来创建一个自适应的窗口界面。首先,需要导入 Tkinter 库并创建一个顶层窗口:
```python
import tkinter as tk
root = tk.Tk()
root.title('自适应窗口界面')
```
然后,可以使用 Tkinter 的布局管理器来创建界面布局。可以使用 `grid` 布局管理器将界面分成两列,再在中间分布三个按钮。
首先,创建两个文本框:
```python
text1 = tk.Text(root, width=40, height=10)
text2 = tk.Text(root, width=40, height=10)
```
接下来,使用 `grid` 布局管理器将文本框放入窗口中:
```python
text1.grid(row=0, column=0)
text2.grid(row=0, column=1)
```
最后,创建三个按钮:
```python
button1 = tk.Button(root, text='按钮1')
button2 = tk.Button(root, text='按钮2')
button3 = tk.Button(root, text='按钮3')
```
并使用 `grid` 布局管理器将按钮放入窗口中:
```python
button1.grid(row=1, column=0)
button2.grid(row=1, column=1)
button3.grid(row=1, column=2)
```
完整的代码如下:
```python
import tkinter as tk
root = tk.Tk()
root.title('自适应窗口界面')
text1 = tk.Text(root, width=40, height=10)
text2 = tk.Text(root, width=40, height=10)
text1.grid(row=0, column=0)
text2.grid(row=0, column=1)
button1 = tk.Button(root, text='按钮1')
button2
### 回答2:
要使用Tkinter创建一个窗口自适应,有两列文本框,中间分布有三个按钮的界面,我们可以按照以下步骤来实现:
首先,导入Tkinter模块,并创建一个窗口对象。然后,使用.pack()方法将窗口自适应布局。
接着,创建两个列的文本框。我们可以使用Tkinter的Entry()方法来创建文本框,并使用.pack()方法将文本框放置在窗口的左右两侧。
```python
import tkinter as tk
window = tk.Tk()
window.pack()
left_textbox = tk.Entry(window)
left_textbox.pack(side='left')
right_textbox = tk.Entry(window)
right_textbox.pack(side='right')
```
然后,创建三个按钮。我们可以使用Tkinter的Button()方法来创建按钮,并使用.pack()方法将按钮放置在窗口的中部。
```python
button1 = tk.Button(window, text='Button 1')
button1.pack()
button2 = tk.Button(window, text='Button 2')
button2.pack()
button3 = tk.Button(window, text='Button 3')
button3.pack()
```
最后,我们使用window.mainloop()来启动窗口的主循环,使窗口保持打开状态。
```python
window.mainloop()
```
这样,我们就成功创建了一个窗口自适应,有两列文本框,中间分布有三个按钮的界面。
### 回答3:
要使用Tkinter创建一个窗口自适应的界面,有两列文本框和三个按钮,可以参考以下代码实现:
```python
import tkinter as tk
def button1_click():
# 按钮1的点击事件处理函数
# TODO: 在这里添加按钮1的点击事件处理逻辑
pass
def button2_click():
# 按钮2的点击事件处理函数
# TODO: 在这里添加按钮2的点击事件处理逻辑
pass
def button3_click():
# 按钮3的点击事件处理函数
# TODO: 在这里添加按钮3的点击事件处理逻辑
pass
root = tk.Tk()
root.title("自适应窗口")
# 创建两列文本框
text1 = tk.Text(root, height=10, width=20)
text1.grid(row=0, column=0, padx=10, pady=10)
text2 = tk.Text(root, height=10, width=20)
text2.grid(row=0, column=1, padx=10, pady=10)
# 创建三个按钮
button1 = tk.Button(root, text="按钮1", command=button1_click)
button1.grid(row=1, column=0, padx=10, pady=10)
button2 = tk.Button(root, text="按钮2", command=button2_click)
button2.grid(row=1, column=1, padx=10, pady=10)
button3 = tk.Button(root, text="按钮3", command=button3_click)
button3.grid(row=1, column=2, padx=10, pady=10)
root.mainloop()
```
以上代码通过Tkinter创建了一个窗口,其中包含了两列文本框和三个按钮。文本框和按钮都采用`grid`布局方式进行排列,并设置了适当的内边距。
当按钮被点击时,会触发相应的点击事件处理函数(`button1_click`、`button2_click`、`button3_click`),你可以在这些函数中添加对应按钮的点击事件处理逻辑。
希望以上的代码能够满足你的需求。如果有任何问题,请随时提问。
帮我用python语言写一个图形化界面,要求有两个显示的窗口和一个执行功能的按键
好的,以下是一个简单的图形化界面的代码,包含两个显示窗口和一个执行功能的按键。你可以根据自己的需求进行修改和完善。
```python
import tkinter as tk
class GUI:
def __init__(self, master):
self.master = master
master.title("图形化界面")
# 创建两个显示窗口
self.window1 = tk.Canvas(master, width=200, height=200, bg="white")
self.window1.pack(side=tk.LEFT)
self.window2 = tk.Canvas(master, width=200, height=200, bg="white")
self.window2.pack(side=tk.RIGHT)
# 创建执行功能的按键
self.button = tk.Button(master, text="执行", command=self.execute)
self.button.pack()
def execute(self):
# 在窗口1中显示执行结果
self.window1.create_text(100, 100, text="执行成功!")
# 在窗口2中显示执行结果
self.window2.create_text(100, 100, text="执行失败!")
if __name__ == '__main__':
root = tk.Tk()
gui = GUI(root)
root.mainloop()
```
在这个例子中,我们使用了tkinter模块创建了一个GUI类,并在该类中定义了两个显示窗口和一个执行功能的按键。在按下执行按钮后,我们在两个窗口中分别显示了不同的执行结果。
阅读全文
相关推荐
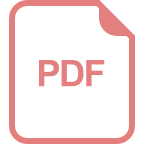
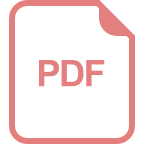
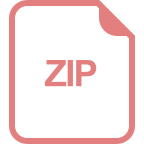













