用HTML寫一個俄羅斯方塊游戲代碼
时间: 2024-03-13 15:48:12 浏览: 14
HTML 是一种标记语言,主要用于网页的布局和呈现,无法直接实现游戏逻辑和交互等方面的功能。如果您想要编写一个完整的俄罗斯方块游戏,需要借助其他编程语言和技术,如 JavaScript、CSS、Canvas 等。下面是一个简单的 HTML、CSS 和 JavaScript 代码示例,可以实现一个基本的俄罗斯方块游戏:
HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>俄罗斯方块游戏</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="game-container">
<canvas id="game-canvas"></canvas>
<div id="game-info">
<h1>俄罗斯方块游戏</h1>
<p>得分:<span id="score">0</span></p>
<p>下一个方块:</p>
<canvas id="next-canvas"></canvas>
<button id="start-button">开始游戏</button>
</div>
</div>
<script src="game.js"></script>
</body>
</html>
```
CSS 代码:
```css
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
background-color: #f5f5f5;
}
#game-container {
display: flex;
align-items: center;
justify-content: center;
height: 100vh;
}
#game-canvas {
background-color: #fff;
border: 2px solid #ccc;
}
#game-info {
margin-left: 50px;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
#game-info h1 {
margin: 0;
font-size: 36px;
}
#game-info p {
margin: 10px 0;
font-size: 24px;
}
#game-info #score {
font-weight: bold;
color: #f00;
}
#game-info #next-canvas {
background-color: #fff;
border: 2px solid #ccc;
margin-top: 10px;
}
#game-info #start-button {
margin-top: 20px;
padding: 10px 20px;
font-size: 24px;
background-color: #f00;
color: #fff;
border: none;
border-radius: 10px;
cursor: pointer;
}
```
JavaScript 代码:
```javascript
// 定义方块类型和颜色
var blockTypes = [
{ type: "I", color: "#00f" },
{ type: "J", color: "#00f" },
{ type: "L", color: "#00f" },
{ type: "O", color: "#ff0" },
{ type: "S", color: "#0f0" },
{ type: "T", color: "#a0a" },
{ type: "Z", color: "#f00" }
];
// 定义方块的形状
var blockShapes = {
"I": [
[0, 0, 0, 0],
[1, 1, 1, 1],
[0, 0, 0, 0],
[0, 0, 0, 0]
],
"J": [
[1, 0, 0],
[1, 1, 1],
[0, 0, 0]
],
"L": [
[0, 0, 1],
[1, 1, 1],
[0, 0, 0]
],
"O": [
[1, 1],
[1, 1]
],
"S": [
[0, 1, 1],
[1, 1, 0],
[0, 0, 0]
],
"T": [
[0, 1, 0],
[1, 1, 1],
[0, 0, 0]
],
"Z": [
[1, 1, 0],
[0, 1, 1],
[0, 0, 0]
]
};
// 获取画布和上下文
var gameCanvas = document.getElementById("game-canvas");
var gameCtx = gameCanvas.getContext("2d");
var nextCanvas = document.getElementById("next-canvas");
var nextCtx = nextCanvas.getContext("2d");
// 定义游戏参数
var blockSize = 20;
var gameWidth = 10;
var gameHeight = 20;
var gameSpeed = 500;
var currentBlock = null;
var nextBlock = null;
var gameBoard = [];
var gameScore = 0;
var gameInterval = null;
var isGameOver = false;
// 初始化游戏面板
for (var i = 0; i < gameHeight; i++) {
gameBoard[i] = [];
for (var j = 0; j < gameWidth; j++) {
gameBoard[i][j] = null;
}
}
// 随机生成下一个方块
function generateNextBlock() {
var index = Math.floor(Math.random() * blockTypes.length);
var blockType = blockTypes[index];
var blockShape = blockShapes[blockType.type];
nextBlock = {
type: blockType.type,
color: blockType.color,
shape: blockShape,
row: 0,
col: Math.floor((gameWidth - blockShape[0].length) / 2)
};
}
// 绘制方块
function drawBlock(block, ctx) {
ctx.fillStyle = block.color;
for (var i = 0; i < block.shape.length; i++) {
for (var j = 0; j < block.shape[i].length; j++) {
if (block.shape[i][j] !== 0) {
ctx.fillRect(block.col * blockSize + j * blockSize, block.row * blockSize + i * blockSize, blockSize, blockSize);
}
}
}
}
// 绘制游戏面板
function drawBoard() {
gameCtx.clearRect(0, 0, gameCanvas.width, gameCanvas.height);
for (var i = 0; i < gameHeight; i++) {
for (var j = 0; j < gameWidth; j++) {
if (gameBoard[i][j] !== null) {
gameCtx.fillStyle = gameBoard[i][j];
gameCtx.fillRect(j * blockSize, i * blockSize, blockSize, blockSize);
}
}
}
}
// 绘制下一个方块
function drawNextBlock() {
nextCtx.clearRect(0, 0, nextCanvas.width, nextCanvas.height);
drawBlock(nextBlock, nextCtx);
}
// 判断方块是否可以移动
function canMove(block, row, col) {
for (var i = 0; i < block.shape.length; i++) {
for (var j = 0; j < block.shape[i].length; j++) {
if (block.shape[i][j] !== 0) {
var newRow = row + i;
var newCol = col + j;
if (newRow < 0 || newRow >= gameHeight || newCol < 0 || newCol >= gameWidth || gameBoard[newRow][newCol] !== null) {
return false;
}
}
}
}
return true;
}
// 将方块加入游戏面板
function addToBoard(block) {
for (var i = 0; i < block.shape.length; i++) {
for (var j = 0; j < block.shape[i].length; j++) {
if (block.shape[i][j] !== 0) {
gameBoard[block.row + i][block.col + j] = block.color;
}
}
}
}
// 消除整行
function clearLines() {
var fullRows = [];
for (var i = 0; i < gameHeight; i++) {
var rowIsFull = true;
for (var j = 0; j < gameWidth; j++) {
if (gameBoard[i][j] === null) {
rowIsFull = false;
break;
}
}
if (rowIsFull) {
fullRows.push(i);
}
}
for (var i = 0; i < fullRows.length; i++) {
var row = fullRows[i];
for (var j = 0; j < gameWidth; j++) {
gameBoard[row][j] = null;
}
for (var k = row - 1; k >= 0; k--) {
for (var l = 0; l < gameWidth; l++) {
gameBoard[k + 1][l] = gameBoard[k][l];
}
}
gameScore += 100;
}
}
// 游戏结束
function gameOver() {
isGameOver = true;
clearInterval(gameInterval);
alert("游戏结束!得分:" + gameScore);
}
// 游戏循环
function gameLoop() {
// 移动方块
currentBlock.row++;
if (canMove(currentBlock, currentBlock.row, currentBlock.col)) {
drawBoard();
drawBlock(currentBlock, gameCtx);
} else {
currentBlock.row--;
addToBoard(currentBlock);
clearLines();
if (currentBlock.row === 0) {
gameOver();
} else {
currentBlock = nextBlock;
generateNextBlock();
drawNextBlock();
}
}
}
// 开始游戏
function startGame() {
if (gameInterval !== null) {
clearInterval(gameInterval);
}
gameScore = 0;
isGameOver = false;
gameInterval = setInterval(gameLoop, gameSpeed);
currentBlock = nextBlock;
generateNextBlock();
drawBoard();
drawBlock(currentBlock, gameCtx);
drawNextBlock();
document.getElementById("score").textContent = gameScore;
}
// 绑定事件
document.getElementById("start-button").addEventListener("click", startGame);
```
这份代码只是一个简单的示例,仅供参考。如果您想要进一步了解俄罗斯方块游戏的实现方式,可以查阅相关的教程和文档。
相关推荐
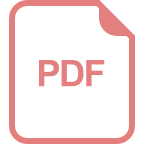
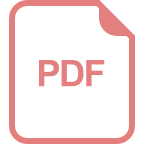










