STN-CNN-LSTM-CTC代码实现
时间: 2023-09-30 08:05:36 浏览: 61
STN-CNN-LSTM-CTC是一种基于卷积神经网络(CNN)、长短时记忆网络(LSTM)、空间变换网络(STN)和CTC(连接时序分类)的端到端语音识别模型。它的实现需要使用深度学习框架,如Tensorflow或PyTorch等。
以下是一个Tensorflow实现的代码示例:
```python
import tensorflow as tf
from tensorflow.contrib.rnn import LSTMCell
# 定义STN网络
def stn(image, theta, out_size):
# 定义一个空间变换网络层
with tf.name_scope('STN'):
# 从theta参数中提取出平移和旋转参数
theta = tf.reshape(theta, (-1, 2, 3))
# 通过theta参数生成变换矩阵
t_g = tf.contrib.image.transform(theta, image, out_size)
return t_g
# 定义CNN网络
def cnn(inputs, is_training):
# 定义卷积层和池化层
conv1 = tf.layers.conv2d(inputs, filters=32, kernel_size=[3, 3], padding='same', activation=tf.nn.relu)
pool1 = tf.layers.max_pooling2d(conv1, pool_size=[2, 2], strides=2)
conv2 = tf.layers.conv2d(pool1, filters=64, kernel_size=[3, 3], padding='same', activation=tf.nn.relu)
pool2 = tf.layers.max_pooling2d(conv2, pool_size=[2, 2], strides=2)
# 展平卷积层输出
shape = pool2.get_shape().as_list()
pool2_flat = tf.reshape(pool2, [-1, shape[1] * shape[2] * shape[3]])
# 定义全连接层
fc1 = tf.layers.dense(pool2_flat, 512, activation=tf.nn.relu)
fc1 = tf.layers.dropout(fc1, rate=0.5, training=is_training)
fc2 = tf.layers.dense(fc1, 512, activation=tf.nn.relu)
fc2 = tf.layers.dropout(fc2, rate=0.5, training=is_training)
return fc2
# 定义LSTM网络
def lstm(inputs, num_layers, num_units):
# 定义多层LSTM网络
cells = []
for i in range(num_layers):
cell = LSTMCell(num_units)
cells.append(cell)
stacked_lstm = tf.contrib.rnn.MultiRNNCell(cells, state_is_tuple=True)
# 运行LSTM网络
outputs, _ = tf.nn.dynamic_rnn(stacked_lstm, inputs, dtype=tf.float32)
return outputs
# 定义CTC网络
def ctc_loss(inputs, targets, seq_length):
# 定义CTC损失函数
ctc_loss = tf.nn.ctc_loss(targets, inputs, seq_length)
loss = tf.reduce_mean(ctc_loss)
return loss
# 定义整个模型
def model(inputs, targets, seq_length, is_training):
# 运行STN网络
theta = cnn(inputs, is_training)
transformed_inputs = stn(inputs, theta, (32, 100))
# 运行CNN网络
cnn_outputs = cnn(transformed_inputs, is_training)
# 运行LSTM网络
lstm_outputs = lstm(cnn_outputs, num_layers=2, num_units=256)
# 定义输出层
logits = tf.layers.dense(lstm_outputs, units=26 + 1) # 26个字母和一个空格
# 定义损失函数
loss = ctc_loss(logits, targets, seq_length)
# 返回输出和损失函数
return logits, loss
```
这个代码实现包括STN网络、CNN网络、LSTM网络和CTC损失函数,可以用于端到端语音识别任务。需要注意的是,这个实现只是一个示例,具体的实现细节可能需要根据具体任务和数据集进行调整。
相关推荐
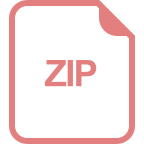
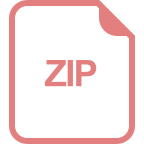
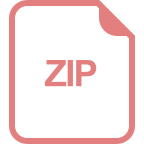














