将mnist数据集转化为图像
时间: 2023-10-09 16:04:42 浏览: 102
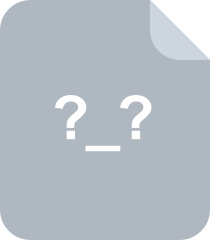
MNIST数据集读取转化为图像
转化mnist数据集为图像需要使用Python中的PIL库和numpy库。你可以使用numpy库加载MNIST数据集,并使用PIL库将数据集转化为图像。
下面是一个简单的代码示例:
```python
import numpy as np
from PIL import Image
def load_mnist(path, kind='train'):
"""Load MNIST data from `path`"""
labels_path = f'{path}/{kind}-labels-idx1-ubyte.gz'
images_path = f'{path}/{kind}-images-idx3-ubyte.gz'
with open(labels_path, 'rb') as lbpath:
magic, n = struct.unpack('>II', lbpath.read(8))
labels = np.frombuffer(lbpath.read(), dtype=np.uint8)
with open(images_path, 'rb') as imgpath:
magic, num, rows, cols = struct.unpack(">IIII", imgpath.read(16))
images = np.frombuffer(imgpath.read(), dtype=np.uint8).reshape(len(labels), 28, 28)
return images, labels
def mnist_to_image(path):
images, labels = load_mnist(path)
for i in range(len(images)):
img = Image.fromarray(images[i], 'L')
img.save(f'{i}.png')
```
在这个代码示例中,`load_mnist`函数使用`struct`模块和numpy库从mnist数据集文件中加载图像和标签。然后,`mnist_to_image`函数将每个图像转化为PIL库中的图像对象,并以PNG格式保存,文件名为该图像在数据集中的索引。
你可以使用类似于`mnist_to_image('/path/to/mnist')`的方式调用`mnist_to_image`函数将MNIST数据集转化为图像。
阅读全文
相关推荐
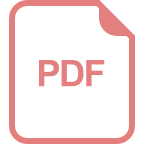
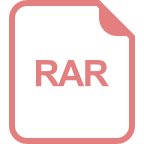
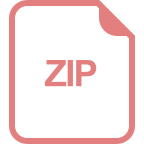
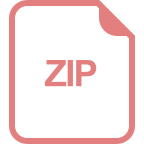
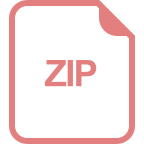
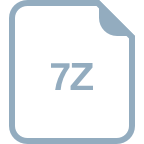
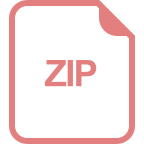
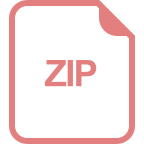
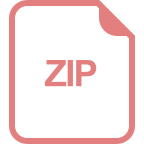
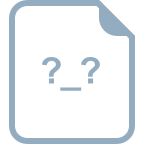
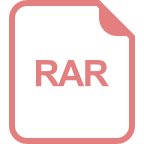
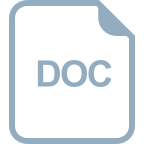


