#include <stdio.h> #include <string.h> #define MAX_STUDENT_NUM 30 // #define MAX_COURSE_NUM 6 // #define NAME_LEN 20 // struct Student { char id[10];//学生学号 char name[NAME_LEN];//姓名 int scores[MAX_COURSE_NUM];//各科分数 int total_score;//总分 float avg_score;//平均分 }; void calc_total_avg_student(struct Student students[], int num, int course_num);//计算每个学生的总分和平均分 void sort_by_total_score(struct Student students[], int num);//按每个学生的总分由高到低排出名次表 void sort_by_number(struct Student students[], int num);//按学号由小到大排出成绩表 请用c语言分别补充函数内容
时间: 2024-01-13 21:03:32 浏览: 125
补充calc_total_avg_student函数内容:
```
void calc_total_avg_student(struct Student students[], int num, int course_num)
{
for (int i = 0; i < num; i++)
{
int total_score = 0;
for (int j = 0; j < course_num; j++)
{
total_score += students[i].scores[j];
}
students[i].total_score = total_score;
students[i].avg_score = (float)total_score/course_num;
}
}
```
补充sort_by_total_score函数内容:
```
void sort_by_total_score(struct Student students[], int num)
{
for (int i = 0; i < num-1; i++)
{
for (int j = 0; j < num-i-1; j++)
{
if (students[j].total_score < students[j+1].total_score)
{
struct Student temp = students[j];
students[j] = students[j+1];
students[j+1] = temp;
}
}
}
}
```
补充sort_by_number函数内容:
```
void sort_by_number(struct Student students[], int num)
{
for (int i = 0; i < num-1; i++)
{
for (int j = 0; j < num-i-1; j++)
{
if (strcmp(students[j].id, students[j+1].id) > 0)
{
struct Student temp = students[j];
students[j] = students[j+1];
students[j+1] = temp;
}
}
}
}
```
注:这里假设MAX_COURSE_NUM为6,NAME_LEN为20。
阅读全文
相关推荐
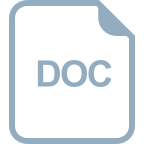
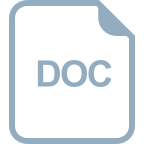
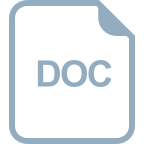









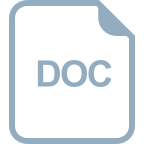
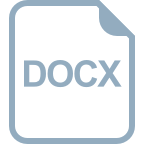
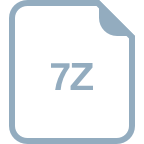