线程安全的map
时间: 2023-07-26 18:20:12 浏览: 63
在多线程编程中,使用普通的map可能会引发线程安全问题,因为多个线程同时对同一个map进行读写操作可能会导致数据不一致的问题。
为了避免这种问题,可以使用线程安全的map。在C++11标准中,引入了std::map的线程安全版本std::map<std::mutex>,通过使用互斥锁来保证并发访问的安全性。例如:
```
#include <map>
#include <mutex>
std::map<int, std::string> my_map;
std::mutex my_mutex;
void add_to_map(int key, const std::string& value) {
std::lock_guard<std::mutex> lock(my_mutex);
my_map[key] = value;
}
std::string get_from_map(int key) {
std::lock_guard<std::mutex> lock(my_mutex);
auto it = my_map.find(key);
if (it != my_map.end()) {
return it->second;
} else {
return "";
}
}
```
在上面的例子中,my_map是一个普通的std::map,而my_mutex是一个互斥锁。add_to_map函数和get_from_map函数都使用了std::lock_guard来保证在访问my_map时,互斥锁已经被获取,从而保证了线程安全性。
除了std::map<std::mutex>之外,还有其他的线程安全map实现,比如Google的ConcurrentHashMap,Boost库中的boost::unordered_map等,可以根据具体需求选择适合的实现。
相关推荐
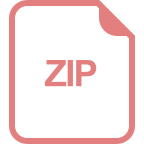














