java声明一个名为person的抽象类,声明共有抽象方法void calsalary(),employee类继承自person类,定义带三个参数的构造方法 String name,int age,String occupation,覆写父类的printinfo(),覆写父类的抽象方法calsalary(),将salary赋值为5000,Worker类继承自person类,内容同employee一样,分别实例化employee类与worker类的对象,并调用各自构造方法初始化属性
时间: 2024-03-25 22:38:41 浏览: 88
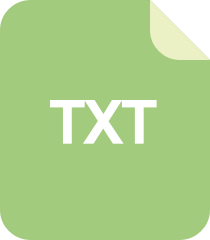
java 类、对象、构造方法的理解
好的,以下是 Java 代码实现:
```java
// 声明抽象类 Person
abstract class Person {
// 声明共有抽象方法 void calsalary()
public abstract void calsalary();
}
// Employee 类继承自 Person 类
class Employee extends Person {
String name;
int age;
String occupation;
int salary;
// 带三个参数的构造方法
public Employee(String name, int age, String occupation) {
this.name = name;
this.age = age;
this.occupation = occupation;
}
// 覆写父类的 printinfo() 方法
public void printinfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.println("Occupation: " + occupation);
System.out.println("Salary: " + salary);
}
// 覆写父类的抽象方法 calsalary()
public void calsalary() {
salary = 5000;
}
}
// Worker 类同样继承自 Person 类
class Worker extends Person {
String name;
int age;
String occupation;
int salary;
// 带三个参数的构造方法
public Worker(String name, int age, String occupation) {
this.name = name;
this.age = age;
this.occupation = occupation;
}
// 覆写父类的 printinfo() 方法
public void printinfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
System.out.println("Occupation: " + occupation);
System.out.println("Salary: " + salary);
}
// 覆写父类的抽象方法 calsalary()
public void calsalary() {
salary = 5000;
}
}
// 实例化 Employee 类与 Worker 类的对象,并调用各自构造方法初始化属性
public class Main {
public static void main(String[] args) {
Employee emp = new Employee("John", 30, "Engineer");
emp.calsalary();
emp.printinfo();
System.out.println();
Worker worker = new Worker("Mary", 25, "Factory worker");
worker.calsalary();
worker.printinfo();
}
}
```
希望这个例子可以帮助你更好地理解 Java 中的抽象类和继承。
阅读全文
相关推荐
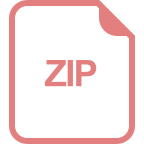
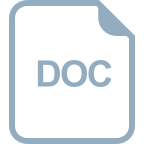
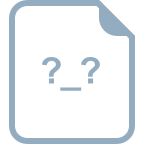
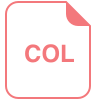









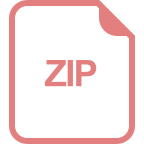