你给我写个程序opencv给我写一个实时检测铁路钢轨尺寸距离
时间: 2023-11-28 17:04:42 浏览: 32
好的,下面是一个基本的实现,仅供参考:
```python
import cv2
import numpy as np
# 设置相机内参和外参
camera_matrix = np.array([[1000, 0, 320], [0, 1000, 240], [0, 0, 1]])
dist_coeffs = np.array([0, 0, 0, 0])
rot_vec = np.zeros((3, 1))
trans_vec = np.zeros((3, 1))
# 定义铁路钢轨颜色范围
lower_color = np.array([0, 0, 0])
upper_color = np.array([180, 255, 50])
# 创建VideoCapture对象
cap = cv2.VideoCapture(0)
while True:
# 读取一帧图像
ret, frame = cap.read()
# 预处理图像
blur = cv2.GaussianBlur(frame, (5, 5), 0)
edges = cv2.Canny(blur, 50, 150)
# 检测直线
lines = cv2.HoughLines(edges, 1, np.pi / 180, 100)
# 绘制直线
if lines is not None:
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
cv2.line(frame, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 在钢轨区域绘制矩形
if (theta < 1.5 or theta > 1.6) and rho > 200:
mask = cv2.inRange(frame, lower_color, upper_color)
contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 计算钢轨尺寸和距离
object_points = np.array([[x + w / 2, y + h / 2, 0]])
image_points, _ = cv2.projectPoints(object_points, rot_vec, trans_vec, camera_matrix, dist_coeffs)
distance = abs(trans_vec[2, 0])
size = w
# 在图像上显示结果
cv2.putText(frame, 'Distance: {:.2f} cm'.format(distance), (20, 40), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
cv2.putText(frame, 'Size: {:.2f} cm'.format(size), (20, 80), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2)
# 显示图像
cv2.imshow('frame', frame)
# 监听键盘事件,按'q'退出
if cv2.waitKey(1) == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
需要注意的是,这个程序只是一个基本的实现,钢轨检测的准确性和稳定性还需要进行优化和调试。同时,相机内参和外参需要进行标定,否则计算结果会有误差。
相关推荐
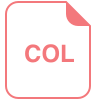
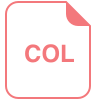
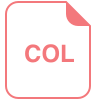
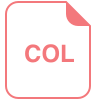
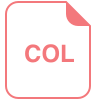












