unity2d实现点击按钮图片轮播
时间: 2023-11-18 11:04:36 浏览: 171
首先需要准备好多张图片,并将它们放入一个文件夹中。
1. 创建一个空场景,并创建一个空物体作为容器,用于存放所有的图片。将容器命名为“Image Container”。
2. 在“Image Container”下创建多个子物体,每个子物体代表一张图片。并将每个子物体的图片设置为对应的图片。
3. 在每张图片的子物体上添加一个Button组件,用于响应点击事件。并将每个Button的事件设置为同一个脚本中的方法。
4. 创建一个脚本,用于实现图片轮播的逻辑。在脚本中定义一个数组,用于存放所有的图片子物体。并定义一个当前图片的索引变量。
5. 在脚本的Start()方法中,获取所有的图片子物体,并将它们存放到数组中。然后将数组中除了第一张图片的子物体全部隐藏。
6. 在脚本中定义一个方法,用于处理按钮点击事件。在该方法中,先将当前图片的子物体隐藏,然后将当前图片的索引加1,如果当前图片的索引超过了数组的长度,则将索引重置为0。最后将下一个图片的子物体显示出来。
7. 将脚本挂载到场景中的任意一个物体上,并将所有的按钮的事件设置为该脚本中的方法。运行场景,点击按钮,可以看到图片轮播的效果。
相关问题
unity2d实现点击按钮图片轮播的具体操作和完整代码
具体操作:
1. 创建一个空的场景,并向场景中添加一个Canvas对象。
2. 在Canvas下创建一个Image对象,作为背景图片,并设置其大小和位置。
3. 在Canvas下创建一个空的GameObject对象,作为容器和管理器。
4. 在容器对象下创建多个Image对象,用于显示轮播的图片,并设置它们的大小和位置。
5. 创建一个Button对象,作为轮播控制按钮,并设置其大小和位置。
6. 在Button对象上添加一个OnClick事件,并指定其对应的函数。
7. 在代码中实现轮播逻辑,即通过按钮点击来切换图片的显示。
完整代码:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ImageCarousel : MonoBehaviour
{
public Image[] images; // 存储所有轮播图片
public Button nextButton; // 下一张按钮
public Button prevButton; // 上一张按钮
private int currentIndex; // 当前显示的图片索引
// Start is called before the first frame update
void Start()
{
// 初始化索引值和显示状态
currentIndex = 0;
UpdateImageDisplay();
// 添加按钮点击事件
nextButton.onClick.AddListener(NextImage);
prevButton.onClick.AddListener(PrevImage);
}
// 切换到下一张图片
void NextImage()
{
currentIndex++;
if (currentIndex >= images.Length)
{
currentIndex = 0;
}
UpdateImageDisplay();
}
// 切换到上一张图片
void PrevImage()
{
currentIndex--;
if (currentIndex < 0)
{
currentIndex = images.Length - 1;
}
UpdateImageDisplay();
}
// 更新图片显示状态
void UpdateImageDisplay()
{
for (int i = 0; i < images.Length; i++)
{
if (i == currentIndex)
{
images[i].gameObject.SetActive(true);
}
else
{
images[i].gameObject.SetActive(false);
}
}
}
}
```
unity2d实现章节图片轮播,点击切换章节的具体操作与代码
1. 在Unity中创建一个新的场景,用于展示章节图片轮播。
2. 创建一个空物体,命名为“ChapterManager”,用于管理章节图片轮播的逻辑。
3. 在ChapterManager中添加一个Image组件,用于展示章节图片。
4. 创建一个数组,用于存储所有的章节图片。
```
public Sprite[] chapterImages;
```
5. 在Start()函数中初始化章节图片数组,并设置初始展示的章节图片。
```
void Start ()
{
chapterImages = Resources.LoadAll<Sprite>("ChapterImages");
currentChapterIndex = 0;
SetChapterImage();
}
```
6. 创建一个整型变量,用于记录当前展示的章节图片的索引值。
```
private int currentChapterIndex;
```
7. 创建两个按钮,分别用于切换到上一章节和下一章节。为按钮添加点击事件,实现切换章节的逻辑。
```
public void PreviousChapter()
{
if (currentChapterIndex > 0)
{
currentChapterIndex--;
}
else
{
currentChapterIndex = chapterImages.Length - 1;
}
SetChapterImage();
}
public void NextChapter()
{
if (currentChapterIndex < chapterImages.Length - 1)
{
currentChapterIndex++;
}
else
{
currentChapterIndex = 0;
}
SetChapterImage();
}
```
8. 创建一个函数,用于设置当前展示的章节图片。
```
private void SetChapterImage()
{
chapterImage.sprite = chapterImages[currentChapterIndex];
}
```
9. 在Unity中创建两个按钮,分别用于触发PreviousChapter()和NextChapter()函数。
10. 将ChapterManager挂载到场景中,并将章节图片数组拖拽到chapterImages变量中。
11. 运行场景,点击按钮实现章节图片轮播的功能。
阅读全文
相关推荐
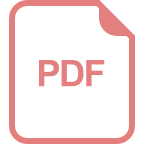












