optional.get without ispresent
时间: 2023-04-26 08:01:05 浏览: 171
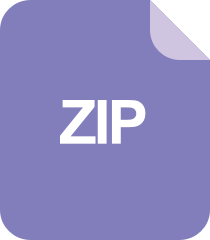
Optional.js:JS的Java可选
optional.get()方法在Optional对象中没有值时会抛出NoSuchElementException异常。因此,应该在调用get()方法之前先使用isPresent()方法检查Optional对象是否有值。如果Optional对象没有值,则可以使用orElse()或orElseGet()方法提供默认值或执行其他操作。
阅读全文
相关推荐

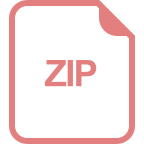
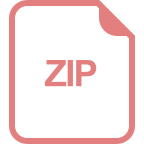
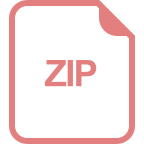
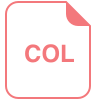

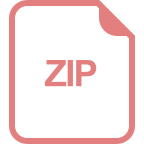
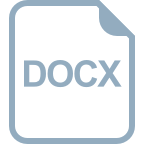
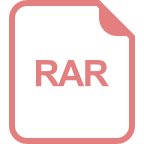
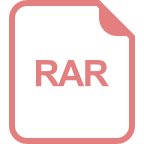
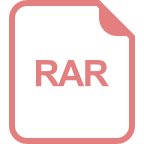
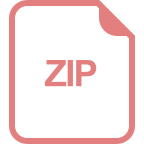
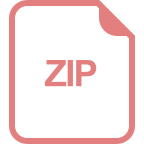
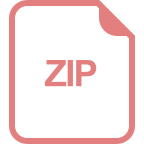