php编写使用正则表达式验证邮箱中用户输入的密码和邮箱和手机号,并实现表单的注册功能
时间: 2024-02-12 13:09:15 浏览: 25
首先,我们可以使用 PHP 的正则表达式函数 `preg_match()` 来验证邮箱、手机号和密码的合法性。下面是一个示例代码:
```php
$email_regex = "/^\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*$/"; // 邮箱正则表达式
$phone_regex = "/^1[3456789]\d{9}$/"; // 手机号正则表达式
$password_regex = "/^[a-zA-Z0-9!@#$%^&*()_+]{6,20}$/"; // 密码正则表达式
$email = $_POST['email'];
$phone = $_POST['phone'];
$password = $_POST['password'];
if (!preg_match($email_regex, $email)) {
// 邮箱格式不正确
echo "邮箱格式不正确";
} else if (!preg_match($phone_regex, $phone)) {
// 手机号格式不正确
echo "手机号格式不正确";
} else if (!preg_match($password_regex, $password)) {
// 密码格式不正确
echo "密码格式不正确";
} else {
// 验证通过,进行注册操作
// ...
}
```
关于表单的注册功能,我们可以使用 HTML 的 `<form>` 元素和 PHP 的 `$_POST` 变量来实现。下面是一个示例代码:
```html
<form action="register.php" method="post">
<label>邮箱:</label>
<input type="text" name="email"><br>
<label>手机号:</label>
<input type="text" name="phone"><br>
<label>密码:</label>
<input type="password" name="password"><br>
<input type="submit" value="注册">
</form>
```
在 `register.php` 文件中,我们可以获取表单提交的数据,进行验证和注册操作。下面是一个示例代码:
```php
$email_regex = "/^\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*$/"; // 邮箱正则表达式
$phone_regex = "/^1[3456789]\d{9}$/"; // 手机号正则表达式
$password_regex = "/^[a-zA-Z0-9!@#$%^&*()_+]{6,20}$/"; // 密码正则表达式
$email = $_POST['email'];
$phone = $_POST['phone'];
$password = $_POST['password'];
if (!preg_match($email_regex, $email)) {
// 邮箱格式不正确
echo "邮箱格式不正确";
} else if (!preg_match($phone_regex, $phone)) {
// 手机号格式不正确
echo "手机号格式不正确";
} else if (!preg_match($password_regex, $password)) {
// 密码格式不正确
echo "密码格式不正确";
} else {
// 验证通过,进行注册操作
// ...
}
```
在注册操作中,我们可以将用户的邮箱、手机号和密码存储到数据库中,以便后续的登录操作。具体的数据库操作可以使用 PHP 的数据库扩展,如 mysqli 或 PDO。
相关推荐
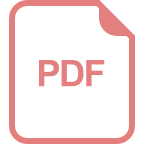
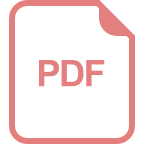
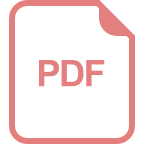














