spring boot整合mybaits plus 页面渲染值
时间: 2023-07-31 14:10:49 浏览: 105
如果你想要在Spring Boot中整合Mybatis Plus,并且在页面中渲染值,你可以按照以下步骤进行:
1. 配置Mybatis Plus
在Spring Boot项目中使用Mybatis Plus,需要在application.properties 或 application.yml文件中添加mybatis-plus的配置,例如:
```
mybatis-plus.mapper-locations=classpath*:mapper/**/*.xml
mybatis-plus.type-aliases-package=com.example.demo.entity
```
其中`mapper-locations`指定了mapper文件(xml)的位置,`type-aliases-package`指定了实体类的包名。
2. 配置Controller
编写Controller类,使用`@RequestMapping`注解来映射请求路径,例如:
```
@Controller
public class UserController {
@Autowired
private UserService userService;
@RequestMapping("/user")
public String user(Model model) {
List<User> userList = userService.list();
model.addAttribute("userList", userList);
return "user";
}
}
```
在上述代码中,我们使用`@RequestMapping`注解映射路径为`/user`的请求,然后调用`userService.list()`方法获取用户列表,并将其添加到`Model`中。最后,返回`user`字符串,它表示我们将使用名为`user.html`的模板文件来渲染视图。
3. 配置Thymeleaf
在Spring Boot中,我们可以使用Thymeleaf作为模板引擎来渲染视图。在`pom.xml`文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
```
然后,在`application.properties`或`application.yml`文件中添加以下配置:
```
spring.thymeleaf.cache=false
spring.thymeleaf.prefix=classpath:/templates/
spring.thymeleaf.suffix=.html
spring.thymeleaf.mode=HTML5
```
其中,`spring.thymeleaf.prefix`指定了模板文件的位置,`spring.thymeleaf.suffix`指定了模板文件的后缀名。
4. 编写模板文件
在`src/main/resources/templates`目录下,创建一个名为`user.html`的文件,并编写以下代码:
```
<!DOCTYPE html>
<html>
<head>
<title>User List</title>
</head>
<body>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr th:each="user : ${userList}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.age}"></td>
</tr>
</tbody>
</table>
</body>
</html>
```
在上述代码中,我们使用Thymeleaf的语法来渲染用户列表。`th:each`指令用于遍历`userList`列表,并将每个用户的ID、姓名和年龄显示在表格中。
5. 运行程序
现在,我们可以启动程序并访问`http://localhost:8080/user`来查看用户列表。如果一切正常,你应该能够看到一个包含用户信息的表格。
希望以上步骤可以帮助你整合Mybatis Plus和Thymeleaf,在Spring Boot中渲染页面。
阅读全文
相关推荐
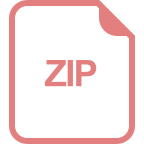
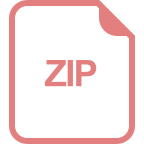
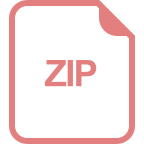
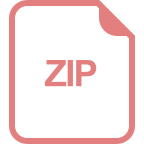
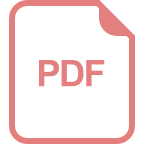
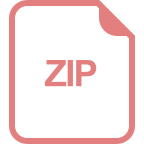
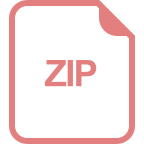
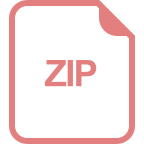
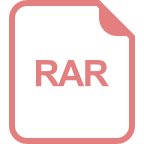
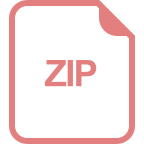
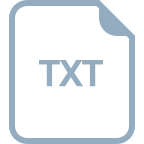
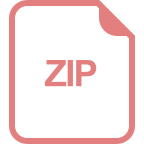
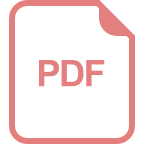
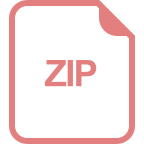

