Attention-BiLSTM
时间: 2023-06-30 07:02:39 浏览: 70
Attention-BiLSTM 是一种深度学习模型,主要应用于自然语言处理领域中的文本分类、情感分析、机器翻译等任务中。它结合了 Attention 机制和双向 LSTM 模型,能够在输入序列中捕捉到双向上下文信息,并且通过 Attention 机制可以对输入序列中的重要信息进行加权,从而提高模型的准确率和泛化能力。
在 Attention-BiLSTM 模型中,首先将输入序列经过双向 LSTM 模型进行编码,得到一个双向上下文感知的隐藏状态序列。然后,通过 Attention 机制计算出每个时间步上输入序列中的权重,将这些权重与编码后的隐藏状态序列进行加权求和,得到一个加权后的表示向量,表示整个输入序列的重要信息。最后,将这个加权后的表示向量送入全连接层进行分类或者生成等任务。
Attention-BiLSTM 模型具有较好的性能,在多个自然语言处理任务中都取得了不错的效果。
相关问题
Attention-BiLSTM的Python代码
下面是一个简单的示例代码,展示了如何在Python中实现Attention-BiLSTM模型:
```python
import tensorflow as tf
from tensorflow.keras.layers import Bidirectional, LSTM, Dense, Embedding, Concatenate, Dot
from tensorflow.keras import Model
class AttentionBiLSTM(Model):
def __init__(self, vocab_size, embedding_dim, hidden_units):
super(AttentionBiLSTM, self).__init__()
self.embedding = Embedding(vocab_size, embedding_dim)
self.bilstm = Bidirectional(LSTM(hidden_units, return_sequences=True))
self.attention = Dot(axes=(1, 1))
self.fc = Dense(1, activation='sigmoid')
def call(self, inputs):
x = self.embedding(inputs)
x = self.bilstm(x)
attention_weights = self.attention([x, x])
context_vector = tf.reduce_sum(attention_weights * x, axis=1)
output = self.fc(context_vector)
return output
```
这个代码定义了一个名为AttentionBiLSTM的自定义模型,包含了嵌入层(Embedding)、双向LSTM层(Bidirectional LSTM)、注意力层(Dot)和全连接层(Dense)。在call方法中,输入数据经过嵌入层和双向LSTM层后,进行注意力计算,并将注意力权重与LSTM输出进行加权求和得到上下文向量。最后,通过全连接层输出预测结果。
请注意,这只是一个参考示例代码,实际使用时可能需要根据具体任务进行修改和调整。
Bert-bilstm-attention
Bert-BiLSTM-Attention是一种常见的文本分类模型,主要由三个部分组成:Bert编码器,BiLSTM编码器和Attention机制。
首先,输入的文本序列经过Bert编码器,得到每个token对应的向量表示。这些向量表示包含了丰富的上下文信息和语义信息,可以作为文本序列的特征表示。
然后,BiLSTM编码器将Bert编码器的输出作为输入,得到每个token在上下文中的向量表示。BiLSTM可以更好地捕捉文本序列中的长期依赖关系,同时也可以防止过拟合。
最后,通过Attention机制,将BiLSTM编码器的输出进行加权平均,得到文本序列的固定长度向量表示,用于文本分类任务。
Attention机制可以根据不同的任务需求,对BiLSTM编码器的输出进行不同的加权,从而突出不同部分的信息。在文本分类任务中,通常使用self-attention机制,即根据BiLSTM编码器的输出,计算每个token与整个文本序列的相似度,然后根据相似度对每个token进行加权平均,得到文本序列的向量表示。
Bert-BiLSTM-Attention模型结构复杂,参数量较大,但在文本分类等自然语言处理任务中,通常可以获得较好的效果。
相关推荐
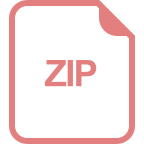
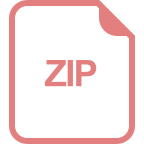
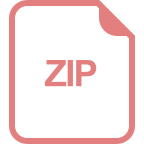
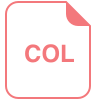
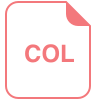
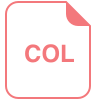
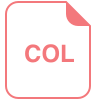
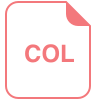







