std::string可替换
时间: 2023-11-16 18:06:40 浏览: 46
好的,我明白了。关于std::string的替代,有很多选择,比如:
1. 使用C风格的字符串(char*);
2. 使用C++11引入的std::wstring;
3. 使用第三方库,比如boost::string或者folly::fbstring等。
但是需要注意的是,这些替代方案都有各自的优缺点,需要根据具体情况进行选择。
相关问题
std::string
`std::string` 是 C++ 标准库中的一个字符串类,使用该类可以方便的进行字符串的操作,如拼接、查找、替换等。
`std::string` 类的头文件为 `<string>`。在使用时,需要包含该头文件,并使用 `using namespace std;`,或者显式地使用 `std::string`。
下面是一些常用的 `std::string` 操作:
1. 创建字符串
```c++
std::string str("hello");
```
2. 访问字符串
```c++
char ch = str[0]; // 访问第一个字符
std::cout << str << std::endl; // 输出字符串
```
3. 拼接字符串
```c++
std::string str1 = "hello";
std::string str2 = "world";
std::string str3 = str1 + " " + str2; // 拼接字符串
```
4. 查找字符串
```c++
std::string str = "hello world";
int pos = str.find("world"); // 查找子串的位置
```
5. 替换字符串
```c++
std::string str = "hello world";
str.replace(0, 5, "hi"); // 将前 5 个字符替换为 "hi"
```
6. 插入字符串
```c++
std::string str = "hello world";
str.insert(5, "cruel "); // 在第 5 个字符处插入字符串
```
7. 删除字符串
```c++
std::string str = "hello world";
str.erase(5, 6); // 从第 5 个字符开始删除 6 个字符
```
以上是一些常用的 `std::string` 操作,更多使用方法可参考官方文档。
介绍一下std::string
std::string是C++ STL库中的一个字符串类,它可以存储和操作任何类型的字符串,包括ASCII和Unicode字符串。
std::string类的特点包括:
1. 动态内存管理:std::string类使用动态内存管理,可以自动调整内存大小以适应字符串的大小变化。
2. 操作方便:std::string类提供了一系列方便的成员函数,包括字符串拼接、截取、替换、查找等操作。
3. 可移植性:std::string类是C++标准库的一部分,可以在各种操作系统和平台上使用,具有良好的可移植性。
4. 安全性:std::string类内部实现了字符串长度的检查,避免了缓冲区溢出等安全问题。
使用std::string类可以简化字符串处理的代码,提高程序的可读性和可维护性。
相关推荐
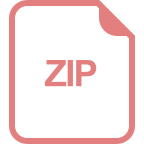
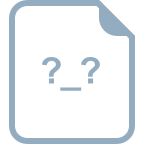
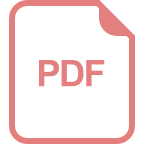












