(1)(考察知识点DBCP数据源) 应用DBCP数据源,实现获取数据库连接对象的功能,并实现增删改查任务代码的编写。 (2)(考察知识点Druid数据源) 使用Druid数据源,实现获取数据库连接对象的功能,并实现增删改查任务代码的编写。 代码:
时间: 2024-02-29 20:55:25 浏览: 39
以下是您需要的代码实现:
使用DBCP数据源:
```
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
public class DBCPExample {
// 获取数据库连接对象
public static Connection getConnection() throws SQLException, NamingException {
Context context = new InitialContext();
DataSource dataSource = (DataSource) context.lookup("java:comp/env/jdbc/testdb");
Connection connection = dataSource.getConnection();
return connection;
}
// 实现增删改查操作
public static void insert() throws SQLException, NamingException {
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = getConnection();
String sql = "insert into user(id, name) values(?, ?)";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setInt(1, 1);
preparedStatement.setString(2, "张三");
int result = preparedStatement.executeUpdate();
System.out.println("插入成功:" + result);
} finally {
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
}
}
public static void delete() throws SQLException, NamingException {
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = getConnection();
String sql = "delete from user where id = ?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setInt(1, 1);
int result = preparedStatement.executeUpdate();
System.out.println("删除成功:" + result);
} finally {
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
}
}
public static void update() throws SQLException, NamingException {
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = getConnection();
String sql = "update user set name = ? where id = ?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, "李四");
preparedStatement.setInt(2, 1);
int result = preparedStatement.executeUpdate();
System.out.println("更新成功:" + result);
} finally {
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
}
}
public static void select() throws SQLException, NamingException {
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
try {
connection = getConnection();
String sql = "select * from user";
preparedStatement = connection.prepareStatement(sql);
resultSet = preparedStatement.executeQuery();
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
System.out.println("id:" + id + ",name:" + name);
}
} finally {
if (resultSet != null) {
resultSet.close();
}
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
}
}
}
```
使用Druid数据源:
```
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
import com.alibaba.druid.pool.DruidDataSourceFactory;
public class DruidExample {
private static DataSource dataSource;
static {
try {
Context context = new InitialContext();
dataSource = (DataSource) context.lookup("java:comp/env/jdbc/testdb");
// 或者使用以下方式获取数据源
// Properties properties = new Properties();
// InputStream inputStream = DruidExample.class.getClassLoader().getResourceAsStream("druid.properties");
// properties.load(inputStream);
// dataSource = DruidDataSourceFactory.createDataSource(properties);
} catch (NamingException e) {
e.printStackTrace();
}
}
// 获取数据库连接对象
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
// 实现增删改查操作
public static void insert() throws SQLException {
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = getConnection();
String sql = "insert into user(id, name) values(?, ?)";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setInt(1, 1);
preparedStatement.setString(2, "张三");
int result = preparedStatement.executeUpdate();
System.out.println("插入成功:" + result);
} finally {
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
}
}
public static void delete() throws SQLException {
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = getConnection();
String sql = "delete from user where id = ?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setInt(1, 1);
int result = preparedStatement.executeUpdate();
System.out.println("删除成功:" + result);
} finally {
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
}
}
public static void update() throws SQLException {
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = getConnection();
String sql = "update user set name = ? where id = ?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, "李四");
preparedStatement.setInt(2, 1);
int result = preparedStatement.executeUpdate();
System.out.println("更新成功:" + result);
} finally {
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
}
}
public static void select() throws SQLException {
Connection connection = null;
PreparedStatement preparedStatement = null;
ResultSet resultSet = null;
try {
connection = getConnection();
String sql = "select * from user";
preparedStatement = connection.prepareStatement(sql);
resultSet = preparedStatement.executeQuery();
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
System.out.println("id:" + id + ",name:" + name);
}
} finally {
if (resultSet != null) {
resultSet.close();
}
if (preparedStatement != null) {
preparedStatement.close();
}
if (connection != null) {
connection.close();
}
}
}
}
```
请将以上代码保存在对应的Java文件中,并将DBCP和Druid相关配置文件(如context.xml和druid.properties)配置好后,部署到Tomcat服务器中。调用相应的方法,即可实现增删改查操作。
相关推荐
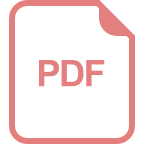
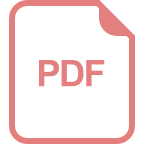
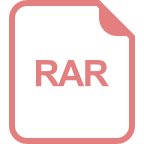















