python中创建一个数据库,每当我将一个文件拖动到pyqt5框架创建的可视化界面后,软件会将文件的地址存入到数据库
时间: 2024-03-16 20:44:55 浏览: 58
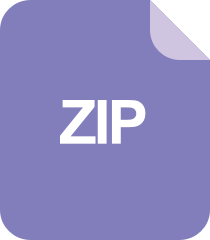
pyqt5 Python 桌面应用程序源码 带数据库
好的,你可以使用Python中的SQLite数据库来实现这个功能。首先,你需要在Python中使用SQLite3模块来连接到数据库并创建一个表来存储文件地址。以下是一个简单的示例代码:
```python
import sqlite3
# 连接到数据库
conn = sqlite3.connect('file_database.db')
# 创建一个表来存储文件地址
conn.execute('''CREATE TABLE IF NOT EXISTS files
(ID INTEGER PRIMARY KEY AUTOINCREMENT,
file_path TEXT NOT NULL);''')
# 关闭连接
conn.close()
```
接下来,你需要在你的PyQt5应用程序中实现拖放操作。你可以使用QDropEvent来获取拖放的文件路径,并将其插入到数据库中。以下是一个示例代码:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QFileDialog
from PyQt5.QtGui import QDropEvent
import os
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setAcceptDrops(True)
def dragEnterEvent(self, event: QDropEvent):
if event.mimeData().hasUrls():
event.accept()
else:
event.ignore()
def dropEvent(self, event: QDropEvent):
for url in event.mimeData().urls():
file_path = str(url.toLocalFile())
# 将文件路径插入到数据库中
conn = sqlite3.connect('file_database.db')
conn.execute("INSERT INTO files (file_path) VALUES ('{}')".format(file_path))
conn.commit()
conn.close()
if __name__ == '__main__':
app = QApplication([])
win = MainWindow()
win.show()
app.exec_()
```
在上面的代码中,我们通过覆盖dragEnterEvent()和dropEvent()方法来实现拖放操作。在dropEvent()方法中,我们首先获取拖放文件的路径,然后将其插入到我们之前创建的数据库表中。
希望这个示例对你有所帮助!
阅读全文
相关推荐

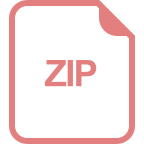
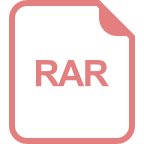
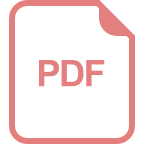
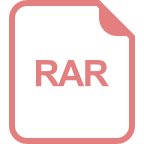
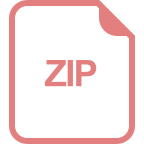
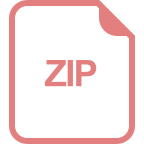
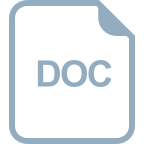
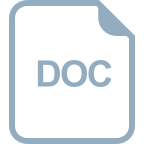
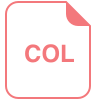
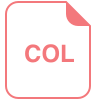
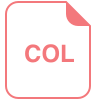

