java爬取cnvd漏洞
时间: 2023-11-04 12:55:11 浏览: 52
要爬取CNVD漏洞信息,可以使用Java中的HttpClient和Jsoup库来实现。具体步骤如下:
1. 使用HttpClient发送请求,获取CNVD漏洞列表页面的HTML源码。
```java
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet("http://www.cnvd.org.cn/flaw/list.htm");
CloseableHttpResponse response = httpClient.execute(httpGet);
String html = EntityUtils.toString(response.getEntity(), "UTF-8");
```
2. 使用Jsoup解析HTML源码,获取漏洞列表。
```java
Document doc = Jsoup.parse(html);
Elements flawList = doc.select(".flaw_list tr:gt(0)");
for (Element flaw : flawList) {
// 获取漏洞信息,如漏洞名称、CNVD-ID、公开日期、危害级别等
String name = flaw.select(".flaw_tit a").text();
String cnvdId = flaw.select(".flaw_list_c td:eq(1)").text();
String publicDate = flaw.select(".flaw_list_c td:eq(2)").text();
String severity = flaw.select(".flaw_list_c td:eq(3)").text();
// TODO: 处理漏洞信息
}
```
3. 可以将获取到的漏洞信息存储到数据库或者文件中。
完整代码示例:
```java
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import java.io.IOException;
public class CnvdCrawler {
public static void main(String[] args) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet("http://www.cnvd.org.cn/flaw/list.htm");
CloseableHttpResponse response = httpClient.execute(httpGet);
String html = EntityUtils.toString(response.getEntity(), "UTF-8");
Document doc = Jsoup.parse(html);
Elements flawList = doc.select(".flaw_list tr:gt(0)");
for (Element flaw : flawList) {
String name = flaw.select(".flaw_tit a").text();
String cnvdId = flaw.select(".flaw_list_c td:eq(1)").text();
String publicDate = flaw.select(".flaw_list_c td:eq(2)").text();
String severity = flaw.select(".flaw_list_c td:eq(3)").text();
System.out.println(name + " " + cnvdId + " " + publicDate + " " + severity);
}
}
}
```
相关推荐
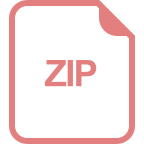
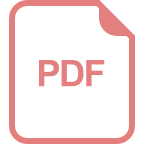














