请解读以下代码:import java.io.Console import response; import request; import http; import java.io.File; import java.io.IOException; import java.nio.file.Files; import com.alibaba.fastjson.JSONObject; import org.apache.commons.codec.binary.Base64; import java.net.URLEncoder; //推送聚会 var juhuiAPIUrl = "http://10.210.26.65:8080/juhuiapi/plugins/notification_service/push_notification?resource=juhui&sysCode=netfault&empNos=%s&msgContent=%s" let itemSubject = "【網絡故障診斷系統】:" + user_name + "主管," + "【網絡故障診斷系統】有待處理事件,請知悉,謝謝!"; let juhuiParams = { "type": "web", "host": "com.foxconn.netfault", "port": "", "path": "", "title": "netfault", "subTitle": itemSubject, "innerurl": "", "outterurl": "", "param": {} } String base64URLSafeString = Base64.encodeBase64URLSafeString(JSONObject.toJSONString(juhuiParams).getBytes("utf-8")); String base64UrlencodeMsg = URLEncoder.encode(base64URLSafeString, "utf-8"); let juhuiResponse = http.connect(String.format(juhuiAPIUrl, userAccount, base64UrlencodeMsg)).post().getBody();
时间: 2023-09-07 20:14:07 浏览: 127
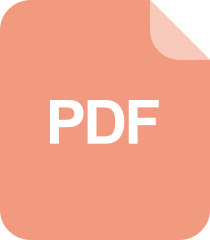
import与export在node.js中的使用详解
这段代码是一个推送聚会(JuHui)通知的功能实现。其中导入了多个Java类库和第三方库,包括Java标准库的Console、File、IOException、URLEncoder、以及第三方库fastjson和commons-codec等。代码中定义了一个推送聚会通知的API地址juhuiAPIUrl,使用了字符串格式化函数String.format(),将userAccount和base64UrlencodeMsg作为参数填充到API地址中,实现了动态构建API地址的功能。
其中,juhuiParams是一个JSON对象,包含了推送通知所需要的各种参数,例如通知的类型、主机、端口、路径、标题、副标题等。使用fastjson库将JSON对象转换成JSON字符串,再使用Base64编码和URL编码,最终得到一个URL安全的Base64编码字符串base64UrlencodeMsg。最后,使用http库中的connect()、post()和getBody()方法,向juhuiAPIUrl发送POST请求,推送聚会通知。
阅读全文
相关推荐
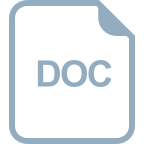
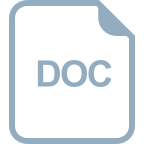



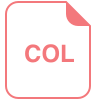
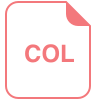
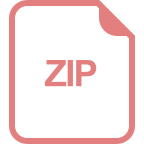
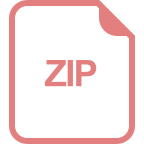
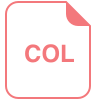
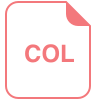
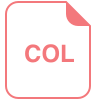
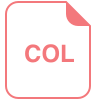
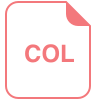
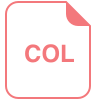
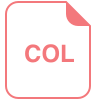
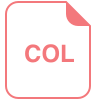
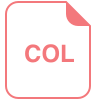
