unity 通过Texture2D刷3D地图可以行走区域 笔刷的形式 最后存入byte数组1代码可行走0代表不可行走,定义地图宽长代码实现
时间: 2024-03-10 09:48:03 浏览: 164
好的,下面是一个示例代码实现,可以通过 `Texture2D` 刷3D地图的行走区域,然后将结果存入一个 `byte` 数组中,其中 1 代表可行走,0 代表不可行走。
```csharp
using UnityEngine;
public class MapEditor : MonoBehaviour
{
public Texture2D mapTexture;
public int brushSize = 1;
public Color brushColor = Color.white;
public int mapWidth = 10;
public int mapHeight = 10;
private byte[,] walkableMap;
private void Awake()
{
walkableMap = new byte[mapWidth, mapHeight];
Color[] mapColors = mapTexture.GetPixels();
for (int y = 0; y < mapHeight; y++)
{
for (int x = 0; x < mapWidth; x++)
{
Color color = mapColors[y * mapWidth + x];
if (color == brushColor)
{
walkableMap[x, y] = 1;
}
}
}
}
private void Update()
{
if (Input.GetMouseButton(0))
{
Vector2 mousePosition = Input.mousePosition;
int x = (int)(mousePosition.x / Screen.width * mapWidth);
int y = (int)(mousePosition.y / Screen.height * mapHeight);
BrushMap(x, y);
}
}
private void BrushMap(int x, int y)
{
for (int i = -brushSize; i <= brushSize; i++)
{
for (int j = -brushSize; j <= brushSize; j++)
{
int px = x + i;
int py = y + j;
if (px >= 0 && px < mapWidth && py >= 0 && py < mapHeight)
{
walkableMap[px, py] = 1;
}
}
}
}
public byte[] GetWalkableMapBytes()
{
byte[] bytes = new byte[mapWidth * mapHeight];
for (int y = 0; y < mapHeight; y++)
{
for (int x = 0; x < mapWidth; x++)
{
bytes[y * mapWidth + x] = walkableMap[x, y];
}
}
return bytes;
}
}
```
上面的代码中,我们首先定义了一个二维的 `byte` 数组来表示地图的行走区域,然后在 `Awake()` 方法中,我们获取了地图纹理中的所有像素点颜色,遍历每一个像素点,如果其颜色与笔刷颜色相同,就将对应的 `walkableMap` 数组中的值设为 1,表示可行走。
在 `Update()` 方法中,我们检测鼠标左键是否被按下,如果是的话,就获取当前鼠标的位置,计算出对应的像素点坐标,然后调用 `BrushMap()` 方法来绘制笔刷。
在 `BrushMap()` 方法中,我们和之前的示例一样,首先使用两个嵌套循环来遍历笔刷的大小,然后根据当前像素点坐标以及笔刷的大小计算出需要修改的地图坐标。注意,我们需要判断计算出来的坐标是否在地图范围内,如果在的话,就将对应的 `walkableMap` 数组中的值设为 1,表示可行走。
最后,我们提供了一个 `GetWalkableMapBytes()` 方法,用于将 `walkableMap` 数组转换为一个一维的 `byte` 数组,并返回给调用者。注意,我们是按行优先的方式来存储地图数据的,即先存储第一行的数据,再存储第二行的数据,以此类推。
上述代码仅供参考,您可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
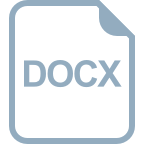
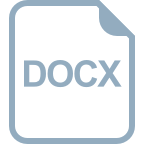
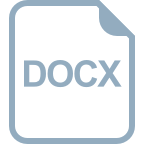
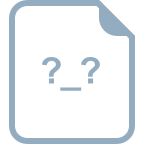
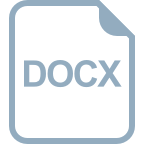
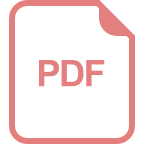
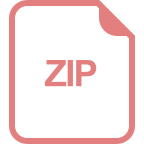
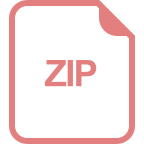
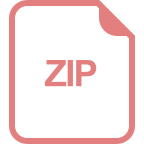
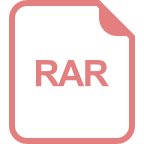
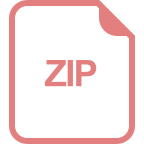
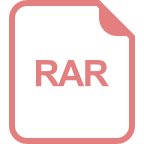
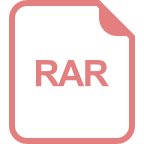
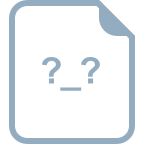