public class TestDataConvert { public static void main(String[] args) { int i=1, j; float f1=0.1; i = i * 0.1; float f2=1234; long s2=88888888888; double d1 = 1e20, d2=125; byte b1 = 10, b2 = 12, b3 = 131; j = j+10; byte b = b1+b2; } }
时间: 2024-01-13 07:03:03 浏览: 109
这段代码中存在一些类型转换错误和未初始化变量错误。具体来说:
1. `float f1=0.1;` 中的 0.1 是一个 double 类型的字面量,需要强制转换为 float 类型,即 `float f1 = (float)0.1;`
2. `i = i * 0.1;` 中的 0.1 同样是 double 类型的字面量,需要强制转换为 int 类型,即 `i = (int)(i * 0.1);`
3. `float f2=1234;` 中的 1234 是 int 类型的字面量,需要强制转换为 float 类型,即 `float f2 = (float)1234;`
4. `long s2=88888888888;` 中的 88888888888 超出了 int 类型的取值范围,需要在末尾加上 L 表示 long 类型的字面量,即 `long s2 = 88888888888L;`
5. `double d1 = 1e20;` 中的 1e20 是科学计数法表示的 double 类型的字面量,无需转换。
6. `byte b1 = 10, b2 = 12, b3 = 131;` 中的 131 超出了 byte 类型的取值范围,需要强制转换为 byte 类型,即 `byte b3 = (byte)131;`
7. `int i=1, j;` 中的 j 未初始化,需要先给 j 赋一个初始值。
8. `j = j+10;` 中的 j 仍然未初始化,需要先给 j 赋一个初始值。
9. `byte b = b1+b2;` 中的 b1 和 b2 都是 byte 类型,相加之后会自动提升为 int 类型,需要强制转换为 byte 类型,即 `byte b = (byte)(b1 + b2);`
正确的代码应该是这样的:
```
public class TestDataConvert {
public static void main(String[] args) {
int i = 1, j = 0;
float f1 = (float)0.1;
i = (int)(i * 0.1);
float f2 = (float)1234;
long s2 = 88888888888L;
double d1 = 1e20, d2 = 125;
byte b1 = 10, b2 = 12, b3 = (byte)131;
j = j + 10;
byte b = (byte)(b1 + b2);
}
}
```
相关推荐
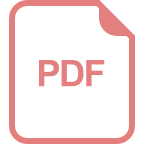
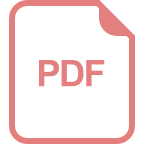
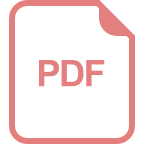















