用c++删除文件夹的指定文档
时间: 2023-09-20 19:04:35 浏览: 50
要删除指定文件夹中的指定文档,可以使用以下代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <filesystem>
namespace fs = std::filesystem;
void deleteFile(const fs::path& folderPath, const std::string& fileName) {
for (auto& file : fs::directory_iterator(folderPath)) {
if (file.is_directory()) {
deleteFile(file.path(), fileName);
} else if (file.path().filename() == fileName) {
std::cout << "Deleting file: " << file.path().string() << std::endl;
fs::remove(file.path());
}
}
}
int main() {
std::string folderPathStr = "path/to/folder";
std::string fileName = "file.txt";
fs::path folderPath(folderPathStr);
if (!fs::exists(folderPath)) {
std::cerr << "Folder does not exist!" << std::endl;
return 1;
}
if (!fs::is_directory(folderPath)) {
std::cerr << "Path is not a folder!" << std::endl;
return 1;
}
deleteFile(folderPath, fileName);
return 0;
}
```
这个程序将递归遍历指定的文件夹,查找所有与指定文件名匹配的文件,并删除它们。注意,如果文件夹中有子文件夹,程序也会递归删除子文件夹中的匹配文件。
相关推荐
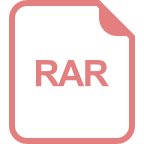
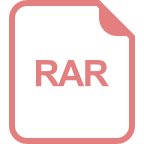
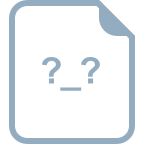














