#include <iostream> using namespace std; int main() { int num1, num2, sum; cout << "请输入第一个数字:"; cin >> num1; cout << "请输入第二个数字:"; cin >> num2; sum = num1 + num2; cout << "两个数的和为:\033[1;36m" << sum << "\033[0m" << endl; return 0; }
时间: 2024-04-28 22:26:22 浏览: 14
这是一个简单的 C++ 程序,它从用户那里获取两个数字,计算它们的和,然后将结果打印到屏幕上。您可以按照以下步骤在 C-Free 5.0 中运行这个程序:
1. 打开 C-Free 5.0 软件,点击 "文件" 菜单,选择 "新建",然后选择 "C++源文件",创建一个新的 C++ 源文件。
2. 将上述代码复制到新建的 C++ 源文件中。
3. 点击 "运行" 菜单,选择 "编译",等待编译完成。
4. 如果编译无误,则点击 "运行" 菜单,选择 "运行",即可在控制台上看到程序的输出结果。
5. 输入第一个数字,按下回车键,再输入第二个数字,按下回车键,程序将会计算这两个数字的和,并将结果输出到屏幕上。
希望这些步骤能够帮助您成功运行这个程序。
相关问题
对于20位以上的超长整数无法用int、long等基本类型表示,但可以考虑用字符串实现。 本关任务:编写程序,基于字符串实现对超长整数的加法运算和减法运算,输入两个超长整数和运算符(+或-),输出运算结果。include <iostream> #include <cstring> using namespace std;
好的,我可以帮你实现这个功能。我们可以使用字符串代替超长整数进行运算,具体实现方法和前面的示例类似,也是逐位相加或逐位相减,并考虑进位和借位的情况。下面是一个简单的实现示例,你可以根据自己的需求进行修改和优化。
```
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
string add(string num1, string num2) {
reverse(num1.begin(), num1.end());
reverse(num2.begin(), num2.end());
int len1 = num1.size(), len2 = num2.size();
int len = max(len1, len2);
int carry = 0;
string res = "";
for (int i = 0; i < len; i++) {
int x = i < len1 ? num1[i] - '0' : 0;
int y = i < len2 ? num2[i] - '0' : 0;
int sum = x + y + carry;
res += to_string(sum % 10);
carry = sum / 10;
}
if (carry) res += to_string(carry);
reverse(res.begin(), res.end());
return res;
}
string sub(string num1, string num2) {
if (num1 == num2) return "0";
bool neg = false;
if (num1.size() < num2.size() || (num1.size() == num2.size() && num1 < num2)) {
swap(num1, num2);
neg = true;
}
reverse(num1.begin(), num1.end());
reverse(num2.begin(), num2.end());
int len1 = num1.size(), len2 = num2.size();
int len = max(len1, len2);
int carry = 0;
string res = "";
for (int i = 0; i < len; i++) {
int x = i < len1 ? num1[i] - '0' : 0;
int y = i < len2 ? num2[i] - '0' : 0;
int diff = x - y - carry;
if (diff < 0) {
diff += 10;
carry = 1;
} else {
carry = 0;
}
res += to_string(diff);
}
reverse(res.begin(), res.end());
while (res.size() > 1 && res[0] == '0') {
res.erase(0, 1);
}
return neg ? "-" + res : res;
}
int main() {
string num1, num2, op;
cin >> num1 >> op >> num2;
if (op == "+") {
cout << add(num1, num2) << endl;
} else if (op == "-") {
cout << sub(num1, num2) << endl;
} else {
cout << "Invalid operator" << endl;
}
return 0;
}
```
#include <iostream>using namespace std;class Calculator { private: int num1, num2; public: Calculator(int n1, int n2) { num1 = n1; num2 = n2; } int add() { return num1 + num2; }};int main() { int n1, n2; cout << "Enter two numbers:" << endl; cin >> n1 >> n2; Calculator calc(n1, n2); cout << "Sum is: " << calc.add() << endl; return 0;}
这是一个简单的 C++ 程序,实现了一个 Calculator(计算器)类,可以对两个整数进行加法运算。在程序中,用户会被要求输入两个整数,然后创建一个 Calculator 对象,并调用其 add 方法,将计算结果输出到屏幕上。程序中的 class 关键字用于定义类,private 关键字用于声明私有成员变量和方法,public 关键字用于声明公共成员变量和方法。在构造函数中,使用了初始化列表的方式对成员变量进行初始化。
相关推荐
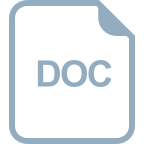
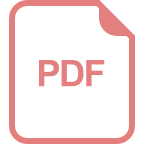
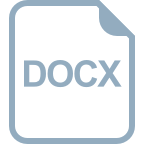













