keras.utils.sequence
时间: 2023-04-26 08:01:18 浏览: 214
keras.utils.sequence是Keras中的一个模块,用于生成序列数据的工具。它提供了一些类和函数,可以帮助我们将数据转换为适合于训练模型的格式。例如,我们可以使用Sequence类来生成批量数据,或者使用pad_sequences函数来将序列填充到相同的长度。这些工具可以帮助我们更方便地处理序列数据,提高模型的训练效率和准确率。
相关问题
keras.utils.Sequence
Keras.utils.Sequence is a base class used for creating custom sequence data generators for training deep learning models in Keras. It is used to efficiently load and preprocess large datasets that might not fit entirely into memory.
A sequence is a type of generator that provides an efficient way to iterate over a large dataset. It is used to feed data to a model in batches during training, validation, or testing. The Sequence class provides an implementation of this generator that ensures thread-safe and ordered data loading.
The Sequence class requires the implementation of two methods: __getitem__ and __len__. The __getitem__ method is responsible for returning a batch of data given an index. The __len__ method returns the total number of batches in the sequence.
To create a custom Sequence, you need to subclass the Keras.utils.Sequence class and implement these two methods based on your data format and preprocessing requirements. Once the Sequence is defined, it can be used in the Keras API for training, validation, and testing.
python keras.utils.Sequence
The `keras.utils.Sequence` class is an abstract base class that allows you to create a custom data generator for training neural networks with Keras. It provides a convenient way to load and preprocess large amounts of data directly from disk, without having to keep the entire dataset in memory at once.
To use `Sequence`, you need to subclass it and implement two methods:
- `__len__(self)`: returns the total number of batches in your dataset
- `__getitem__(self, index)`: returns the `index`-th batch of data
You can also optionally implement the `on_epoch_end(self)` method, which is called at the end of each epoch to perform any required actions (e.g., shuffling the data).
Here's an example of how to use `Sequence` to create a custom data generator:
```python
from keras.utils import Sequence
class MyDataGenerator(Sequence):
def __init__(self, data_dir, batch_size):
self.data_dir = data_dir
self.batch_size = batch_size
self.data_files = os.listdir(data_dir)
def __len__(self):
return len(self.data_files) // self.batch_size
def __getitem__(self, index):
batch_files = self.data_files[index*self.batch_size:(index+1)*self.batch_size]
batch_data = []
for file_name in batch_files:
data = load_data(os.path.join(self.data_dir, file_name))
preprocessed_data = preprocess_data(data)
batch_data.append(preprocessed_data)
return np.array(batch_data)
def on_epoch_end(self):
np.random.shuffle(self.data_files)
```
In this example, `MyDataGenerator` takes a directory containing data files and a batch size as input. `__len__` returns the number of batches (i.e., the number of files divided by the batch size), and `__getitem__` loads and preprocesses each batch of data. `on_epoch_end` shuffles the list of data files at the end of each epoch.
Once you've defined your custom data generator, you can use it to train your Keras model like this:
```python
model.fit_generator(generator=MyDataGenerator(data_dir='data/', batch_size=32),
steps_per_epoch=1000,
epochs=10)
```
阅读全文
相关推荐
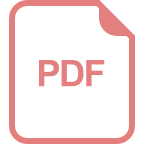







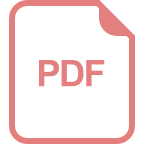




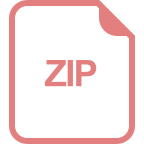