用Python写一个控制安卓手机屏幕的代码
时间: 2024-04-29 18:23:02 浏览: 9
由于安卓手机屏幕控制需要调用安卓系统的API,因此需要使用Python的安卓开发框架——Kivy。
以下是一个简单的示例代码,可以通过按钮来控制手机屏幕的亮度:
```python
from kivymd.app import MDApp
from kivy.uix.boxlayout import BoxLayout
from android.runnable import run_on_ui_thread
from jnius import autoclass
Activity = autoclass('android.app.Activity')
WindowManager = autoclass('android.view.WindowManager$LayoutParams')
LayoutParams = autoclass('android.view.WindowManager$LayoutParams')
class ScreenControlApp(MDApp):
def build(self):
layout = BoxLayout(orientation='vertical')
button_up = MDIconButton(icon='brightness-6', pos_hint={'center_x': 0.5, 'center_y': 0.6})
button_up.bind(on_press=self.increase_brightness)
layout.add_widget(button_up)
button_down = MDIconButton(icon='brightness-3', pos_hint={'center_x': 0.5, 'center_y': 0.4})
button_down.bind(on_press=self.decrease_brightness)
layout.add_widget(button_down)
return layout
@run_on_ui_thread
def increase_brightness(self, *args):
activity = Activity.mActivity
params = activity.getWindow().getAttributes()
params.screenBrightness = WindowManager.BRIGHTNESS_OVERRIDE_FULL
activity.getWindow().setAttributes(params)
@run_on_ui_thread
def decrease_brightness(self, *args):
activity = Activity.mActivity
params = activity.getWindow().getAttributes()
params.screenBrightness = WindowManager.BRIGHTNESS_OVERRIDE_OFF
activity.getWindow().setAttributes(params)
```
在这个示例中,我们使用了Kivy和KivyMD来创建了一个包含两个按钮的垂直布局。当用户点击按钮时,我们使用`run_on_ui_thread`装饰器将代码放在UI线程上执行,然后调用安卓系统的API来控制屏幕亮度。`increase_brightness`函数将屏幕亮度设置为最大值,`decrease_brightness`函数将屏幕亮度设置为最小值。
需要注意的是,在使用这个代码之前需要确保安卓设备已经安装了Kivy和KivyMD,并且在代码运行之前需要先将手机开发者选项中的USB调试选项打开。
相关推荐
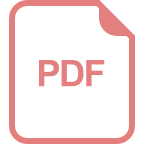
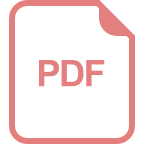
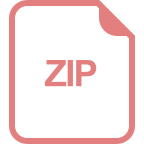














