实现根据ISBN二分查找,返回图书的书名作者出版社
时间: 2023-07-19 09:41:35 浏览: 141
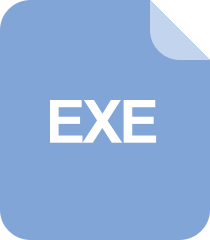
根据图书ISBN读取书名等信息

实现根据ISBN二分查找,返回图书的书名、作者、出版社可以使用已经按ISBN排序的数组,或者使用二叉搜索树来实现。
下面是使用已经按ISBN排序的数组的例子:
```
struct Book {
int id; // 图书编号
char name[50]; // 图书名称
char author[50]; // 作者
char publisher[50]; // 出版社
float price; // 价格
long long isbn; // ISBN编号
};
struct Book books[100]; // 图书数组,假设最多存储100本书
// 二分查找
struct Book* binarySearch(long long isbn, int n) {
int left = 0;
int right = n - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (books[mid].isbn == isbn) {
return &books[mid];
} else if (books[mid].isbn < isbn) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return NULL;
}
// 示例代码
int main() {
// 假设有10本书,按ISBN从小到大排序
books[0] = {1, "C++ Primer", "Stanley B. Lippman", "Addison-Wesley Professional", 59.99, 9780321714114};
books[1] = {2, "Java Concurrency in Practice", "Brian Goetz", "Addison-Wesley Professional", 49.99, 9780321349606};
books[2] = {3, "Effective C++", "Scott Meyers", "Addison-Wesley Professional", 54.99, 9780321334879};
books[3] = {4, "The C Programming Language", "Brian W. Kernighan and Dennis M. Ritchie", "Prentice Hall", 49.99, 9780131103627};
books[4] = {5, "Introduction to Algorithms", "Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein", "MIT Press", 84.99, 9780262033848};
books[5] = {6, "Design Patterns", "Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides", "Addison-Wesley Professional", 59.99, 9780201633610};
books[6] = {7, "Head First Design Patterns", "Eric Freeman and Elisabeth Robson", "O'Reilly Media", 59.99, 9780596007126};
books[7] = {8, "Clean Code", "Robert C. Martin", "Prentice Hall", 44.99, 9780132350884};
books[8] = {9, "Refactoring", "Martin Fowler", "Addison-Wesley Professional", 54.99, 9780201485677};
books[9] = {10, "Code Complete", "Steve McConnell", "Microsoft Press", 49.99, 9780735619678};
// 搜索ISBN为9780321714114的图书
struct Book* book = binarySearch(9780321714114, 10);
if (book != NULL) {
printf("书名:%s\n作者:%s\n出版社:%s\n", book->name, book->author, book->publisher);
} else {
printf("未找到图书\n");
}
return 0;
}
```
这个例子中,使用二分查找来搜索ISBN,如果找到了对应的图书,就返回图书的信息。
如果使用二叉搜索树来实现,可以把ISBN作为节点的关键字,每个节点包含图书的信息。搜索时,从根节点开始,如果搜索的ISBN小于节点的关键字就往左子树搜索,否则往右子树搜索。如果搜索到了对应的节点,就返回节点的信息。
这里就不提供二叉搜索树的具体实现代码了,因为二分查找已经足够简单高效。
阅读全文
相关推荐
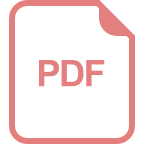
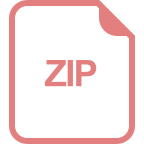
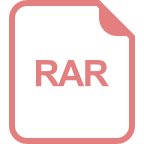
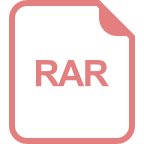
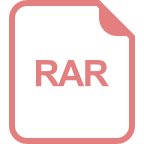
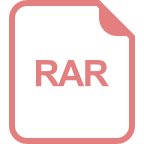
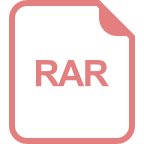
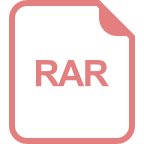
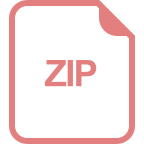
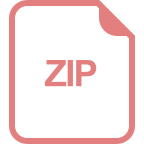
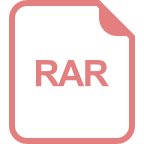
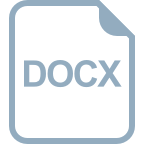
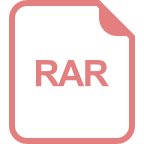
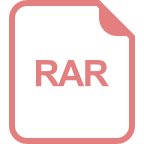
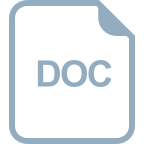
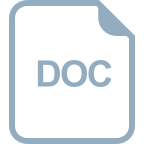
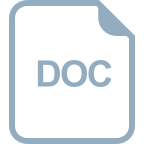
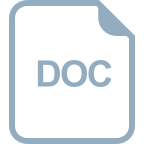