用java代码实现成绩录入界面,要求如下: (1)界面包含两个文本行、一个按钮、一个列表框组件。两个文本行分别输入学生学号和分数,点击录入按钮,学号及分数信息在列表框中显示。 (2)输入成绩范围为0-...
时间: 2023-06-13 10:03:31 浏览: 85
这是一个简单的 Java Swing 界面实现成绩录入的例子,你可以根据自己的需要进行修改:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ScoreEntry extends JFrame {
private JTextField idField, scoreField;
private DefaultListModel<String> listModel;
private JList<String> scoreList;
private JButton addButton;
public ScoreEntry() {
setTitle("成绩录入");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(400, 300);
setLayout(new BorderLayout());
JPanel inputPanel = new JPanel(new GridLayout(2, 2));
JLabel idLabel = new JLabel("学号:");
idField = new JTextField();
JLabel scoreLabel = new JLabel("分数:");
scoreField = new JTextField();
inputPanel.add(idLabel);
inputPanel.add(idField);
inputPanel.add(scoreLabel);
inputPanel.add(scoreField);
add(inputPanel, BorderLayout.NORTH);
JPanel buttonPanel = new JPanel(new FlowLayout());
addButton = new JButton("录入");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String id = idField.getText();
String score = scoreField.getText();
if (!id.isEmpty() && !score.isEmpty()) {
int scoreInt = Integer.parseInt(score);
if (scoreInt >= 0 && scoreInt <= 100) {
String item = id + ":" + score;
listModel.addElement(item);
idField.setText("");
scoreField.setText("");
} else {
JOptionPane.showMessageDialog(ScoreEntry.this, "分数范围应为0-100!");
}
} else {
JOptionPane.showMessageDialog(ScoreEntry.this, "学号和分数不能为空!");
}
}
});
buttonPanel.add(addButton);
add(buttonPanel, BorderLayout.CENTER);
listModel = new DefaultListModel<>();
scoreList = new JList<>(listModel);
JScrollPane scrollPane = new JScrollPane(scoreList);
add(scrollPane, BorderLayout.SOUTH);
setVisible(true);
}
public static void main(String[] args) {
new ScoreEntry();
}
}
```
这个界面包含一个输入面板、一个按钮面板和一个列表框。其中输入面板包含两个文本行,用于输入学号和分数;按钮面板包含一个“录入”按钮,用于将学号和分数记录到列表框中;列表框用于显示所有已录入的学号和分数。
在点击“录入”按钮时,程序会先检查学号和分数是否为空,如果为空则弹出提示框;如果不为空,则将分数转换成整数并检查是否在0-100的范围内,如果不在范围内则弹出提示框;如果在范围内,则将学号和分数组合成一个字符串并添加到列表框中,同时清空输入框。
注意,在这个例子中没有进行输入的合法性检查,如学号是否合法等,你可以根据自己的需要进行修改和完善。
相关推荐
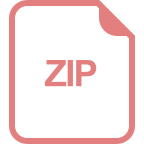
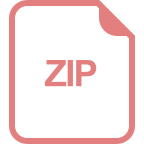
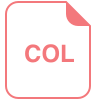
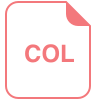
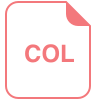
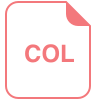
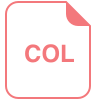









