在不使用库的情况下用javaScript实现HMAC-SHA256
时间: 2024-02-05 14:10:47 浏览: 144
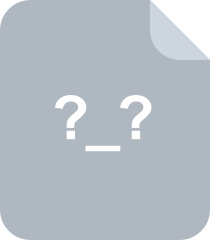
HMAC HmacSHA256校验码生成
HMAC-SHA256是一种哈希算法,它需要密钥和消息输入。在JavaScript中,我们可以使用CryptoJS库来实现HMAC-SHA256。但是,如果您不想使用库,可以尝试以下方法:
```javascript
function hmacSHA256(key, message) {
const sha256HashLength = 64; // SHA-256 hash length in bytes
const blockSize = 512; // HMAC block size in bits
const ipad = 0x36; // Inner padding value
const opad = 0x5c; // Outer padding value
// Convert key and message to byte arrays
const keyBytes = new TextEncoder().encode(key);
const messageBytes = new TextEncoder().encode(message);
// If the key is longer than the block size, hash it first
if (keyBytes.length > blockSize / 8) {
keyBytes = new Uint8Array(await crypto.subtle.digest('SHA-256', keyBytes));
}
// Pad the key with zeros to the block size
const paddedKey = new Uint8Array(blockSize / 8);
paddedKey.set(keyBytes);
paddedKey.forEach((byte, index) => {
paddedKey[index] ^= ipad;
});
// Concatenate the padded key and message
const concatenated = new Uint8Array(paddedKey.length + messageBytes.length);
concatenated.set(paddedKey);
concatenated.set(messageBytes, paddedKey.length);
// Hash the concatenated value
const hashed = new Uint8Array(await crypto.subtle.digest('SHA-256', concatenated));
// Pad the outer key with zeros to the block size
const outerPaddedKey = new Uint8Array(blockSize / 8);
outerPaddedKey.set(keyBytes);
outerPaddedKey.forEach((byte, index) => {
outerPaddedKey[index] ^= opad;
});
// Concatenate the outer padded key and the hashed value
const finalConcatenated = new Uint8Array(outerPaddedKey.length + sha256HashLength);
finalConcatenated.set(outerPaddedKey);
finalConcatenated.set(hashed, outerPaddedKey.length);
// Compute the final hash
const finalHash = await crypto.subtle.digest('SHA-256', finalConcatenated);
// Convert the final hash to a hexadecimal string
return Array.from(new Uint8Array(finalHash))
.map((byte) => byte.toString(16).padStart(2, '0'))
.join('');
}
```
上面的函数接受密钥和消息字符串作为输入,并返回一个十六进制字符串,它是HMAC-SHA256哈希。请注意,此函数使用JavaScript的Web Cryptography API来执行哈希计算。如果您需要在旧版浏览器上运行此代码,则需要使用polyfill来支持Web Cryptography API。
阅读全文
相关推荐
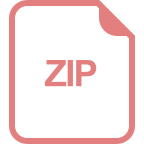




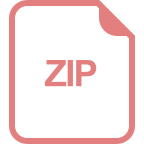
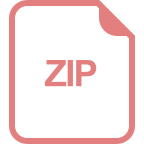
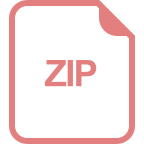
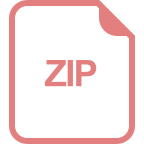
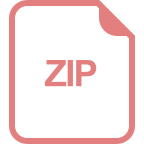
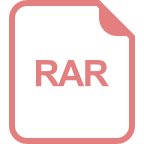
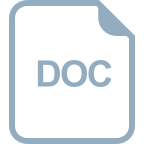




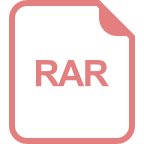